1 year ago
#381231
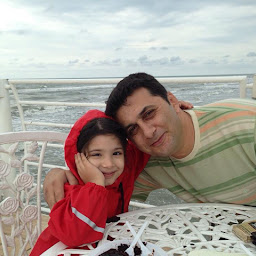
Omid Shojaee
Error while trying to switch the identifier to email in Django User model
I want to change the unique identifier of Django's User model from Username to Email so I write this:
models.py:
from django.db import models
from django.contrib.auth.base_user import BaseUserManager
from django.contrib.auth.models import AbstractUser
# Create your models here.
class CustomUserManager(BaseUserManager):
'''
Custom user model manager where email is the unique identifier for authentication instead of username.'
'''
def create_user(self, email, password, **extra_fields):
'''
Create and save a User with the given email and password.
'''
if not email:
raise ValueError('The Email must be set')
email = self.normalize_email(email)
user = self.model(email=email, **extra_fields)
user.set_password(password)
user.save()
return user
def create_superuser(self, email, password, **extra_fields):
'''
Create and save a SuperUser with the given email and password.
'''
extra_fields.setdefault('is_staff', True)
extra_fields.setdefault('is_superuser', True)
extra_fields.setdefault('is_active', True)
if extra_fields.get('is_staff') is not True:
raise ValueError('Superuser must have is_staff=True.')
if extra_fields.get('is_superuser') is not True:
raise ValueError('Superuser must have is_superuser=True.')
return self.create_user(email, password, **extra_fields)
class CustomUser(AbstractUser):
username = None
email = models.EmailField('email address', unique=True)
USERNAME_FIELD = 'email'
REQUIRED_FIELDS = []
objects = CustomUserManager()
def __str__(self):
return self.email
admin.py:
from django.contrib import admin
from django.contrib.auth.admin import UserAdmin
from .models import CustomUser
# Register your models here.
class CustomUserAdmin(UserAdmin):
model = CustomUser
list_display = ('email', 'is_staff', 'is_active')
list_filter = ('email', 'is_staff', 'is_active')
fieldsets = (
(None, {'fields': ('email', 'password')}),
('Permissions', {'fields': ('is_staff', 'is_active')}),
)
add_fieldsets = (
(
None,
{
'classes': ('wide',),
'fields': ('email', 'password1', 'password2', 'is_staff', 'is_active'),
},
),
)
search_fields = ('email',)
ordering = ('email',)
settings.py:
AUTH_USER_MODEL = 'users.CustomUser'
Both python manage.py makemigrations
and python manage.py migrate
commands completed successfully.
When I run python manage.py createsuperuser
it asks for Email
(which is correct) and then passwords but I get the following after that:
AttributeError: 'CustomUserManager' object has no attribute 'create_superuser'. Did you mean: 'create_user'?
I'm lost.
What is the issue with my code? If there's any other way to do that, would you please give me the link to tutorial?
django
django-authentication
0 Answers
Your Answer