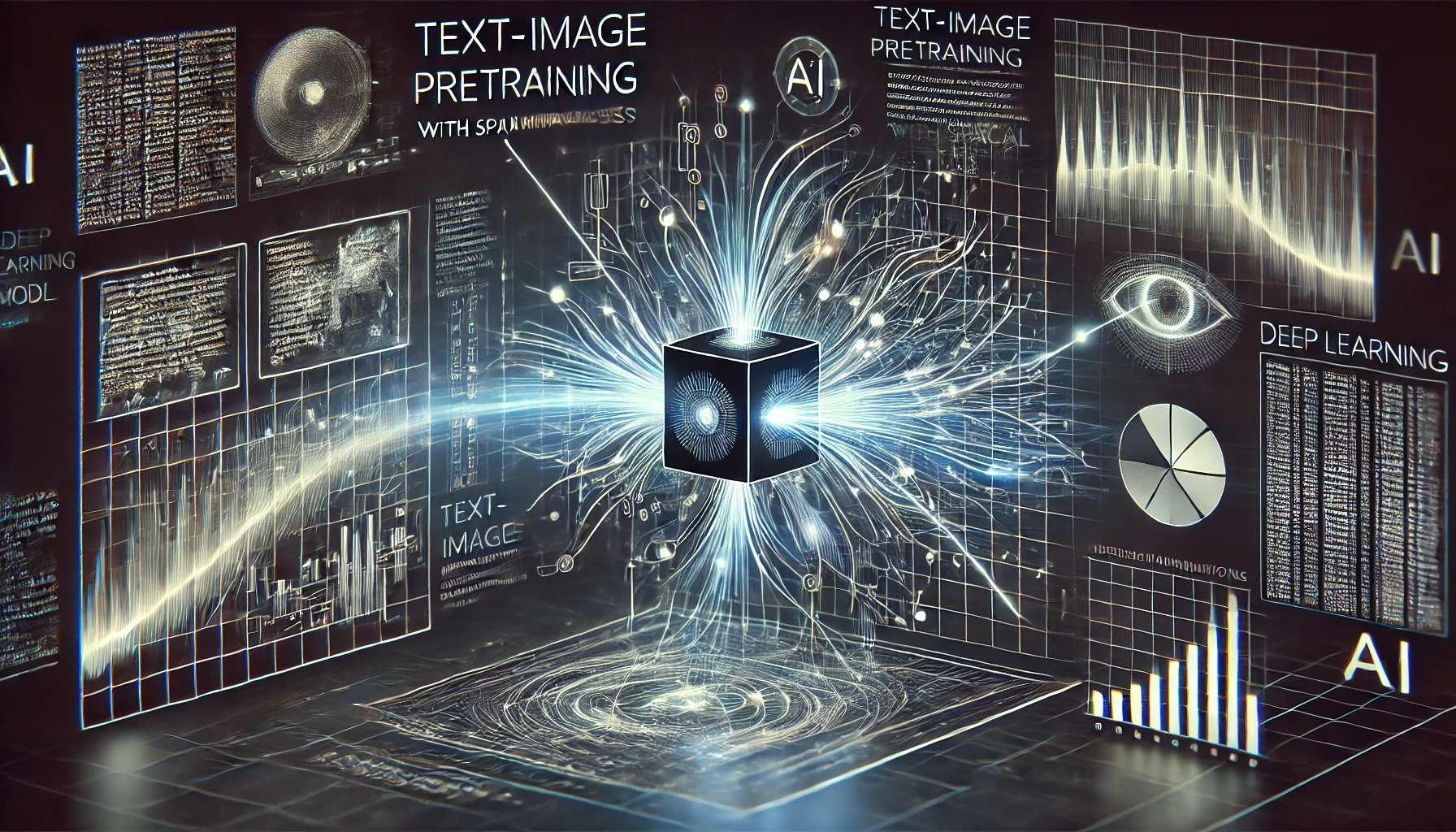
May 01, 2025
TIPS: Unlocking Text-Image Pretraining with Spatial Awareness – A Practical Guide with Code
TIPS: Unlocking Text-Image Pretraining with Spatial Awareness, A Practical Guide with Code
Have you pondered how AI models interpret text-image relationships? How can a model tell that "A cat sitting on a wooden seat" fits an area in an image? In text-image pretraining (TIPS) with spatial awareness, models learn not only what is in an image but also where it is.
Imagine telling a friend about an incident. Not just "There is a dog." Probably, "A dog is sitting beside the tree on the left." This guide explores spatial understanding, which we want AI models to learn.
We will look at how spatial awareness helps AI understand both text and images better. We will also use Python to develop a simple TIPS model with spatial features. Let's start!
Understanding Text-Image Pretraining
Text-image pretraining powers AI models like CLIP, BLIP, and Flamingo that link images with text. These models develop text links by pairing images with descriptive text on enormous datasets.
There is a catch. Most models just show what is in an image, not where. A classic model may match an image of a cat on a table with the words "A cat beneath the table." Spatial awareness helps the AI detect objects in an image, improving its knowledge.
Consider giving the model more intelligence. In addition to understanding what is in an image, the AI learns to detect object relationships. Image captioning, visual search, and scene interpretation need this.
Incorporating Spatial Awareness in TIPS
So, how can we teach AI spatial awareness? Some significant methods:
First, positional encoding lets models track image objects. Giving each pixel a unique coordinate helps the AI locate objects.
Instead of assessing an image as a whole, region-based attention concentrates on specific sections, like human eyes do when studying a situation.
Finally, object detection models like YOLO (You Only Look Once) and Faster R-CNN may help our AI recognize and describe visual objects. Together, these approaches can greatly improve the model's spatial relationship understanding.
Building a Simple TIPS Model with Spatial Features
Okay, enough theory; let's do some coding! We will use Python, PyTorch, and YOLOv8 to build a spatially aware text-image model.
Step 1: Setting Up the Environment
First, install the required libraries:
pip install torch torchvision transformers ultralytics pillow
Let's load a CLIP model that has already been taught to handle our text and images:
import torch
from transformers import CLIPProcessor, CLIPModel
from PIL import Image
# Load CLIP model and processor
model = CLIPModel.from_pretrained("openai/clip-vit-base-patch32")
processor = CLIPProcessor.from_pretrained("openai/clip-vit-base-patch32")
Step 2: Extracting Spatial Features with YOLOv8
We will use YOLOv8 to detect objects in an image and get the dimensions of their bounding boxes.
from ultralytics import YOLO
# Load YOLO model
yolo_model = YOLO("yolov8n.pt")
# Run object detection on an image
results = yolo_model("sample_image.jpg")
# Extract bounding box data
for result in results:
for box in result.boxes:
x1, y1, x2, y2 = box.xyxy[0] # Bounding box coordinates
print(f"Object detected at: ({x1}, {y1}) to ({x2}, {y2})")
The locations of detected objects are now known! We must now integrate this data into our text-image model.
Step 3: Enhancing Text-Image Matching with Spatial Context
We will add spatial coordinates to the CLIP model to calculate similarity scores. Instead of comparing text and image embeddings, we will provide bounding box information.
# Generate image and text embeddings
image_features = model.get_image_features(torch.rand(1, 3, 224, 224)) # Dummy image tensor
text_features = model.get_text_features(["a cat", "a dog"])
# Concatenate spatial coordinates with embeddings
spatial_info = torch.tensor([[x1, y1, x2, y2]]) # Bounding box coordinates
enhanced_features = torch.cat((image_features, spatial_info), dim=1)
print("Enhanced feature vector:", enhanced_features.shape)
Our new model matches text with images and considers object location, enhancing accuracy and relevancy.
Evaluating Model Performance
What proves our spatially-aware model works?
One way is to test it on labeled images with comprehensive annotations from MS COCO or Visual Genome.
Attention maps are another way to do it. They help us see what parts of an image the model is focused on when it makes predictions.
Grad-CAM may highlight image areas that affect model choices. If our model accurately addresses key areas, spatial awareness improves its understanding.
Conclusion
Finally, a realistic way to improve text-image pretraining with spatial awareness!
By adding spatial characteristics, we enable AI models to go beyond text-image matching to better image interpretation. This greatly affects autonomous driving, medical imaging, and AR/VR.
If you want to take this further, try various object detection models or fine-tune CLIP on spatially annotated datasets. There are so many options!
So, what will you develop next?
209 views