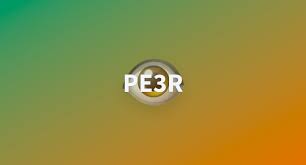
April 30, 2025
PE3R: Mastering Perception-Efficient 3D Reconstruction with Code
PE3R: Mastering Perception-Efficient 3D Reconstruction with Code
Have you ever wondered how video games produce realistic worlds, self-driving vehicles comprehend their surroundings, or AR filters correctly map your face? Reconstructing a 3D representation of the actual environment from images or sensor data is the secret to these technologies.
However, here is a catch: 3D reconstruction is computationally intensive and inefficient. Traditional approaches struggle with occlusions, computing power, and real-world complexity.
PE3R (Perception-Efficient 3D Reconstruction) helps here. PE3R uses depth estimation, point cloud processing, and neural networks to automate the reconstruction pipeline. Best part? Try it without a supercomputer. This article will explain PE3R and show you how to code it so you can easily recreate the world.
Understanding 3D Reconstruction
Before we code, let's clarify: Just what is 3D reconstruction?
3D reconstruction creates a three-dimensional model from 2D photos, videos, or sensor data. Using flat images to create depth, structure, and perspective is reverse engineering.
Unfortunately, standard 3D reconstruction approaches are slow and fuzzy. Many use stereo vision, although it fails in bad lighting or on textureless surfaces. Some use accurate but pricey LiDAR sensors, which are not for everyone.
PE3R handles things differently. Perception efficiency means it collects the most important elements from images and creates a high-quality 3D model without unnecessary calculations. This makes it suitable for real-time gaming, AR, and robotics.
Now it's time for the fun part: coding our own perception-efficient 3D reconstruction framework!
Implementing PE3R: A Step-by-Step Coding Guide
Setting Up the Environment
Install the libraries before we begin. We will use Open3D, OpenCV, and PyTorch for 3D visualization, image processing, and depth estimation.
# Install required libraries
!pip install open3d numpy opencv-python torch torchvision
Now that we have that setup, let's load and preprocess images.
Loading and Preprocessing Images
To rebuild, we need an image. It will help with feature extraction if we load it and change it to grayscale.
import cv2
import numpy as np
# Load an image
img = cv2.imread("sample.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Show the image
cv2.imshow("Grayscale Image", gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
Once we have a preprocessed image, we need to predict how deep it goes to understand how the 3D structure works.
Depth Estimation Using AI
We will not use stereo vision, but instead a depth estimation model based on deep learning. Intel's MiDaS is one of the best lightweight models on the market.
import torch
from torchvision import transforms
from PIL import Image
# Load the MiDaS model for depth estimation
model = torch.hub.load("intel-isl/MiDaS", "MiDaS_small")
model.eval()
def estimate_depth(image_path):
img = Image.open(image_path)
transform = transforms.Compose([transforms.Resize((384, 384)), transforms.ToTensor()])
img_tensor = transform(img).unsqueeze(0)
with torch.no_grad():
depth = model(img_tensor)
return depth.numpy()
depth_map = estimate_depth("sample.jpg")
print("Depth map shape:", depth_map.shape)
Now that we have transformed our image into a depth map, we can see how far away each pixel is from the camera. Let's make a 3D model of this now!
Generating a Point Cloud from Depth Data
A point cloud is just a group of 3D points that show an object or surroundings. This is what makes 3D reconstruction work.
import open3d as o3d
def depth_to_point_cloud(depth_map):
point_cloud = o3d.geometry.PointCloud()
points = np.array([[x, y, depth_map[y, x]] for y in range(depth_map.shape[0]) for x in range(depth_map.shape[1])])
point_cloud.points = o3d.utility.Vector3dVector(points)
return point_cloud
pc = depth_to_point_cloud(depth_map)
o3d.visualization.draw_geometries([pc])
Today, we created a 3D point cloud from a single image that works perfectly! But we can make it even better.
Optimizing PE3R for Speed & Efficiency
We have successfully reconstructed a 3D model again, but efficiency is very important. Here's what we can do to make things better:
- Reduce Noise in Depth Estimation: Using smoothing filters to get rid of noise that is not needed.
- Use Lighter Neural Networks: Picking efficient models like MiDaS_small over bigger ones
- Compress the Point Cloud: Make the Point Cloud smaller by reducing the number of points. This will speed up drawing.
Here's a fast depth map noise filter:
import cv2
def smooth_depth_map(depth_map):
return cv2.GaussianBlur(depth_map, (5, 5), 0)
depth_map_smoothed = smooth_depth_map(depth_map)
This little tweak improves 3D output quality while reducing calculations.
Conclusion: What's Next?
So there you have it! We created a perception-efficient 3D reconstruction system employing AI-driven depth estimation and point cloud processing. But this is only the start. Try real-time video reconstruction, GANs for texture improvement, or transformer-based models for accuracy. PE3R reduces the complexity of 3D reconstruction, which is evolving quickly. No problem; capture, reconstruct, and come up with new ideas. The future of 3D begins!
194 views