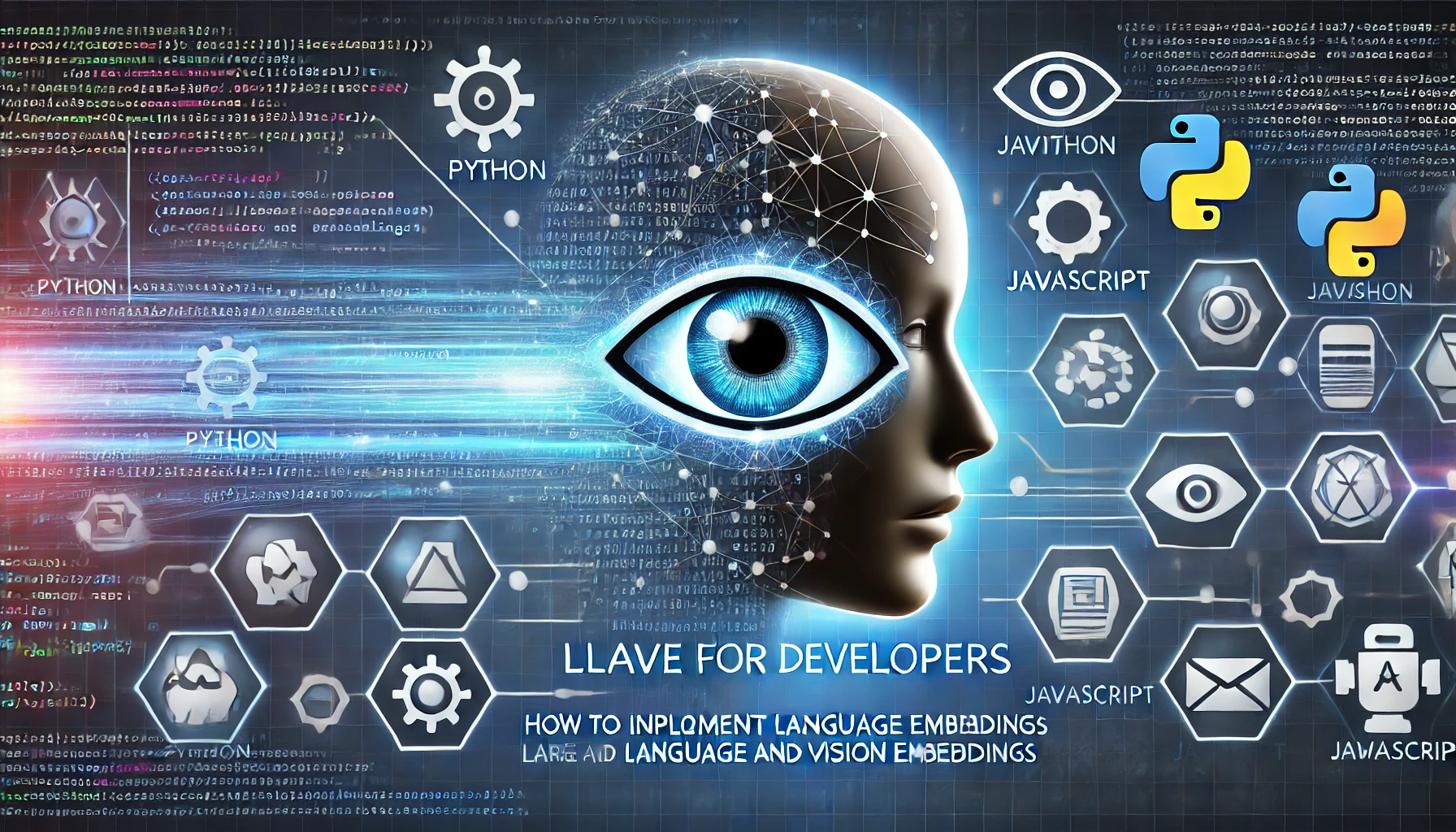
April 28, 2025
LLaVE for Developers: How to Implement Large Language and Vision Embeddings
LLaVE for Developers: How to Implement Large Language and Vision Embeddings
Have you ever wished AI could understand graphics and text both like humans do? Imagine an AI that can analyze a picture, learn its context, and draw conclusions that relate to real-world information. This is what LLaVE seeks to do.
Developers are always pushing AI's limits, and multimodal learning, where AI interprets visuals and text, is the next big thing. LLaVE offers unlimited possibilities for search engines, advanced recommendation systems, and content regulation.
This article explains how to implement LLaVE from scratch. We will build up the environment, develop vision-language embeddings, and fine-tune bespoke task models. No nonsense, just hands-on coding. Ready? Let's jump in!
Understanding LLaVE
Before we code, let's briefly explain LLaVE and why it is so important. Language-focused AI models like GPT or vision-focused ones like ResNet, YOLO, and ViT are typical. However, real-world applications typically need both.
Large Language and Vision Embeddings (LLaVE) creates a shared text-image embedding space to fill this gap. AI can "see" photos and "read" text while creating significant connections. Imagine finding images that match "a cat wearing sunglasses" without labeling them. This is LLaVE's strength!
OpenAI's CLIP (Contrastive Language-Image Pretraining) model is excellent for this job. Multimodal search, imagine captioning, and content tagging benefit from CLIP's ability to match photos to text descriptions. Let's code now!
Setting Up the Environment
Make sure you have Python installed before you start. Then, put in the libraries you need:
pip install torch torchvision transformers sentence-transformers
It is also necessary to have PIL for working with images and the Hugging Face's Transformers library for loading the pre-trained models.
import torch
from transformers import CLIPProcessor, CLIPModel
from PIL import Image
After setting it up, we can start making embeddings.
Generating Vision-Language Embeddings with CLIP
Let's put CLIP to work and make text and image embeddings with it. First, we'll load the model:
model = CLIPModel.from_pretrained("openai/clip-vit-base-patch32")
processor = CLIPProcessor.from_pretrained("openai/clip-vit-base-patch32")
The next step is to make embeddings for an image and some text descriptions:
image = Image.open("sample.jpg") # Replace with your image file
text = ["A dog playing in the park", "A cat sitting on a chair"]
inputs = processor(text=text, images=image, return_tensors="pt", padding=True)
outputs = model(**inputs)
image_embeds = outputs.image_embeds
text_embeds = outputs.text_embeds
At this point, we have successfully taken out embeddings for both the text and the image. What can we do with these embeddings, though? Let's find out.
Using LLaVE for Multimodal Search
Suppose you are making a search engine for pictures. Upload an image to find similar images or text descriptions instead of searching with words.
We can use cosine similarity to compare images to text descriptions using our extracted embeddings:
from torch.nn.functional import cosine_similarity
similarity = cosine_similarity(image_embeds, text_embeds)
print(similarity)
More relevant matches have better similarity scores! Pinterest and Google Lens recommend similar-looking content this way.
Want to go further? With these embeddings in a vector database like FAISS, you can develop a multimodal search engine. The brilliance of LLaVE is its flexibility.
Fine-Tuning LLaVE for Custom Tasks
Pre-trained models perform well, but you may need to customize them for your use case. Medical imaging, fashion items, and satellite images may benefit greatly from fine-tuning.
With Hugging Face's Trainer API, there is an easy way to fine-tune CLIP.
First, define your training dataset:
from datasets import load_dataset
dataset = load_dataset("your_custom_dataset")
Now, set up the training configuration:
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir="./results",
per_device_train_batch_size=8,
evaluation_strategy="epoch",
save_strategy="epoch",
logging_dir="./logs",
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset["train"],
eval_dataset=dataset["test"]
)
trainer.train()
Your model will perform better for domain-specific tasks like medical image classification, e-commerce product labeling, and autonomous driving after fine-tuning.
Conclusion
This is it! We have implemented Large Language and Vision Embeddings (LLaVE) from scratch, generated embeddings using CLIP, and customized a model.
Exciting part? It is only the beginning. In the future, video understanding, 3D multimodal modeling, and real-time AI assistants might "see" and "understand" the world like humans.
If you have come this far, why not try LLaVE in your projects? Build a multimodal chatbot, AI-powered search engine, or content recommendation system. The options are endless!
84 views