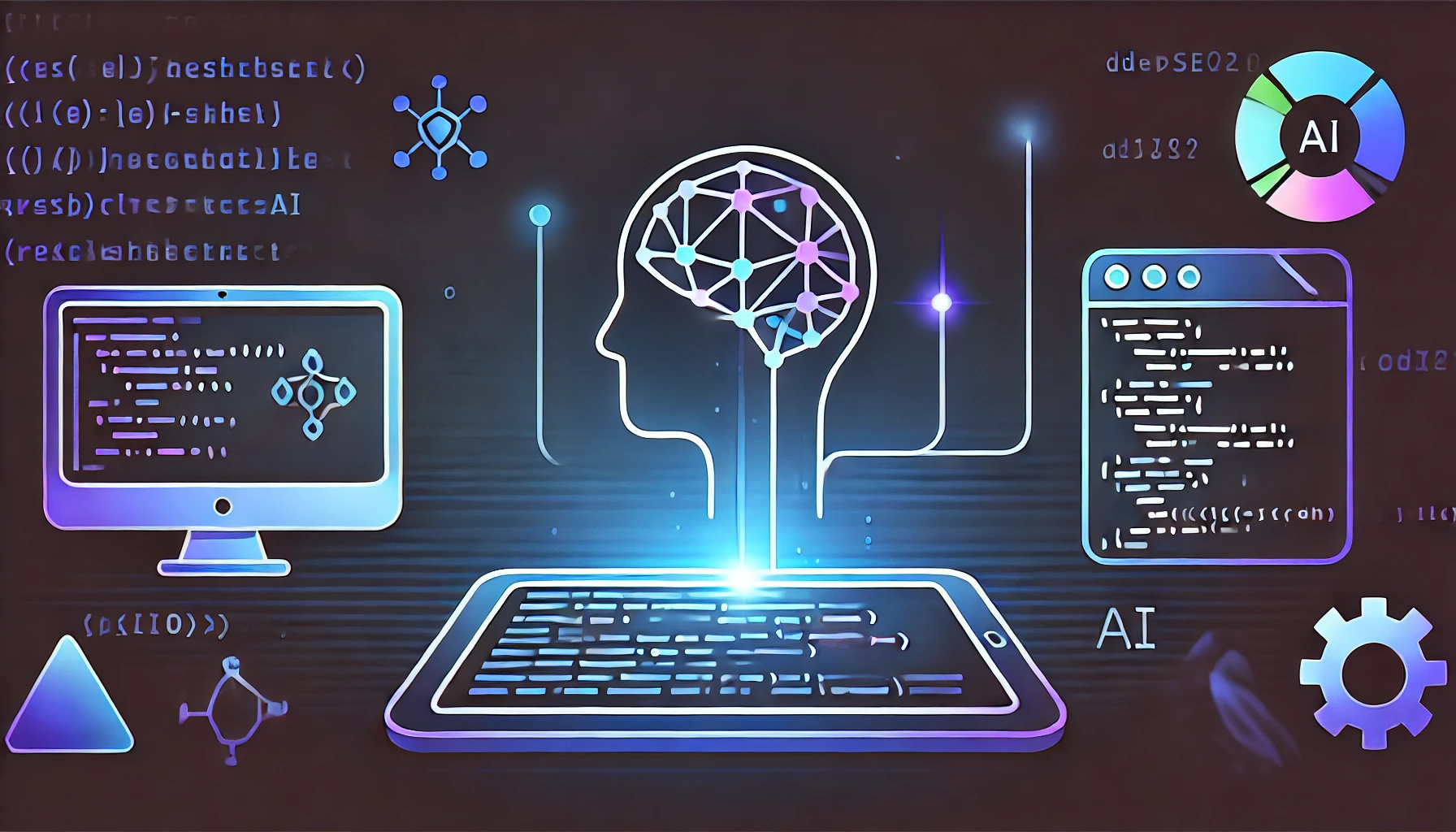
March 28, 2025
Training a DeepSeek Model to Learn Your Codebase
Management of a large codebase is like arranging a large library with new volumes. What was hard? Make sure that developers use the same code style, naming conventions, and best practices. AI-powered DeepSeek generates code and finds flaws without project-specific data. Here comes fine-tuning. You can train DeepSeek on your codebase to create an AI assistant that knows your coding patterns, internal libraries, and architecture. Let's customize DeepSeek to maximize its potential.
Why Fine-Tuning DeepSeek Matters
AI model DeepSeek is strong yet generalist out of the box. Never assume it understands your project's APIs, conventions, or best practices. This might invalidate recommendations or completions. Customizing the model to your codebase improves it.
Consider a large Python project with custom data processing. Generic AI models may misread these functions and propose waste. DeepSeek may learn these patterns to forecast code lines, find issues, and customize documentation to your style.
Model fine-tuning improves collaboration. Since AI teaches new developers to team standards in real time, they onboard quicker. Since DeepSeek finds issues based on your logic rather than generic solutions, debugging is faster.
DeepSeek Fine-Tuning
Before optimizing DeepSeek, prepare your codebase. Structured, high-quality examples help the model learn.
Step 1: Install DeepSeek
To begin, install the required package:
pip install deepseek
Step 2: Load Your Codebase
You need to let DeepSeek access the files in your directory. Use this method to load all project directory python files:
import os
def load_code_from_repo(repo_path):
codebase = []
for root, _, files in os.walk(repo_path):
for file in files:
if file.endswith(".py"): # Load Python files
with open(os.path.join(root, file), "r", encoding="utf-8") as f:
codebase.append(f.read())
return "\n".join(codebase)
# Load project codebase
codebase = load_code_from_repo("my_project/")
print("Loaded codebase with", len(codebase.splitlines()), "lines of code")
Fine-Tuning DeepSeek on a Custom Codebase
To fine-tune, provide DeepSeek with a structured dataset to learn prior coding patterns.
Step 3: Train the AI Model
After loading the code, send it to DeepSeek's fine-tuning module:
from deepseek import DeepSeekFineTuner
# Train DeepSeek on custom codebase
DeepSeekFineTuner.train(codebase)
print("Training complete. Model is now aware of your project's patterns!")
DeepSeek analyzes code to understand features such as function and class name patterns, internal API use, problem solutions, and speed enhancements.
Generating Custom Code with Fine-Tuned DeepSeek
Learning from your codebase, DeepSeek may provide project-specific suggestions.
Step 4: AI-Assisted Code Completion
See how well DeepSeek understands our projects. Imagine our codebase's standard database query mechanism. DeepSeek should create a new function with the same pattern:
query_code = """
def fetch_user_data(user_id: int):
\"\"\"Retrieve user data from the database.\"\"\"
result = db.query(f"SELECT * FROM users WHERE id={user_id}")
return result
"""
response = DeepSeekFineTuner.generate(f"Write a function to fetch order details following this style: {query_code}")
print(response)
DeepSeek will generate:
def fetch_order_data(order_id: int):
\"\"\"Retrieve order data from the database.\"\"\"
result = db.query(f"SELECT * FROM orders WHERE id={order_id}")
return result
Step 5: Debugging with Fine-Tuned DeepSeek
Fine-tuning DeepSeek finds codebase-specific faults, enhancing debugging.
Let's pass in an incorrect function for DeepSeek to fix:
buggy_code = """
def process_payment(amount):
\"\"\"Process a payment transaction.\"\"\"
if amount = 0: # Incorrect condition
return "Invalid amount"
return "Payment processed"
"""
response = DeepSeekFineTuner.generate(f"Fix bugs in this function: {buggy_code}")
print(response)
If amount = 0, DeepSeek accurately detects and corrects the syntax error:
def process_payment(amount):
\"\"\"Process a payment transaction.\"\"\"
if amount == 0: # Fixed condition
return "Invalid amount"
return "Payment processed"
Step 6: Automating API Documentation Generation
Automatic documentation is another DeepSeek fine-tuning use. DeepSeek automates API doc revisions for struggling teams.
function_code = """
def get_user(id: int):
\"\"\"Fetch user details by ID.\"\"\"
return db.get(id)
"""
response = DeepSeekFineTuner.generate(f"Generate API documentation for this function: {function_code}")
print(response)
DeepSeek will output:
### API Endpoint: Get User Details
**Method:** GET
**Description:** Fetch user details by ID.
**Parameters:**
- `id` (int): The user ID
**Response:**
- Returns user data in JSON format
Evaluating and Improving the Fine-Tuned Model
Fine-tuning is only the start. Real-world performance evaluation and model refinement are the magic.
After training, have the model create code in several contexts and compare it to manual code. If DeepSeek still makes terrible recommendations, train it with better examples. DeepSeek receives suggestions via reinforcement learning. Updated and retrained datasets update project needs models.
Automating Continuous Learning
Fine-tune your CI/CD process to improve DeepSeek. How to:
def update_training_data():
new_code = load_code_from_repo("my_project/")
DeepSeekFineTuner.retrain(new_code)
print("Model retrained with latest project updates.")
# Schedule retraining every week
schedule.every().week.do(update_training_data)
This ensures DeepSeek remains aligned with your latest project changes.
Conclusion
DeepSeek model tweaking improves complicated project development. An intelligent coding helper that understands your codebase replaces generic AI. It accelerates development, collaboration, and debugging.
Refined models will become mainstream as AI-powered development grows. Future coding involves writing better code and teaching AI to do it better. Time to improve your development process? Try DeepSeek fine-tuning!
272 views