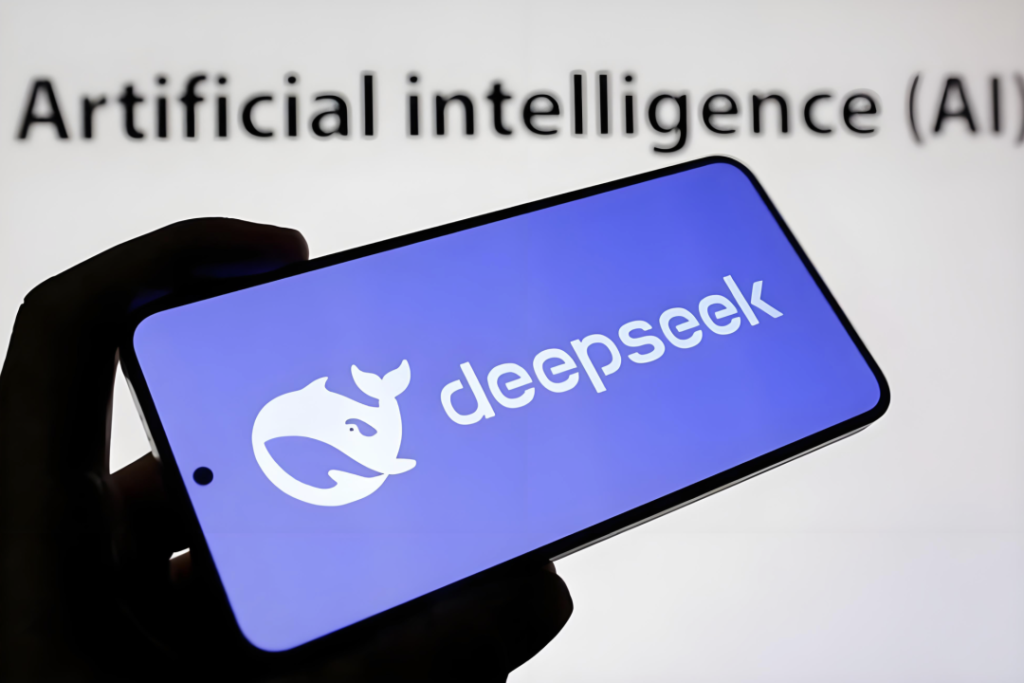
April 02, 2025
Enhancing DeepSeek with Multi-Agent AI Systems
AI is transforming software development. However, one AI model typically provides insufficient, inconsistent, or general input. In my experience, DeepSeek is amazing at evaluating code and recommending changes, but its feedback is occasionally shallow. At that time, I studied multi-agent AI systems, where AI models work together to improve outputs.
Imagine a better AI-powered code review system where DeepSeek analyzes, and GPT-4 adds context-aware recommendations, best practices, and optimization. The result? Enhanced accuracy, performance, and code clarity. Let's develop this multi-agent AI system and explain its game-changing power.
What Are Multi-Agent AI Systems?
Multi-agent AI systems make decisions and solve issues using many AI models. Instead of using a single AI, this strategy allows several models enhance each other for more accurate, reliable, and optimum results.
Why Does This Matter for Code Review?
- AI models that work alone have their limitations. For example, DeepSeek might find problems but miss odd cases.
- GPT-4 is better at understanding bigger patterns, which makes sure that its ideas are in line with best practices for code.
- Working together solves difficulties. Like having several people review code, multiple AI models can improve each other's remarks.
Self-driving vehicles already do this. AI models collaborate to process sensor data, detect objects, and drive. In code review, one AI discovers issues, while another improves the ideas.
Implementing a Multi-Agent AI Code Review System
Building a DeepSeek + GPT-4 multi-agent system that examines Python code and makes recommendations will show this.
Step 1: Setting Up DeepSeek for Initial Code Analysis
DeepSeek effectively detects syntax problems, performance concerns, and security vulnerabilities. First, let us put DeepSeek to review through a simple function:
from deepseek import DeepSeekCode
buggy_code = """
def divide(a, b):
return a / b
"""
deepseek_review = DeepSeekCode.generate(f"Review this code: {buggy_code}")
print(deepseek_review)
Expected Output:
DeepSeek may find a division-by-zero problem but not a way to fix it.
Step 2: Refining DeepSeek's Feedback with GPT-4
We now include GPT-4 as a second AI agent to DeepSeek's suggestions. GPT-4 offers alternate solutions, performance enhancements, and best practices.
from openai import GPT4
def multi_agent_review(code):
deepseek_review = DeepSeekCode.generate(f"Review this code: {code}")
gpt4_feedback = GPT4.chat(f"Improve the DeepSeek review: {deepseek_review}")
return gpt4_feedback
print(multi_agent_review(buggy_code))
Step 3: Implementing the Suggested Fixes
Let's say the AI advises adding division by zero error handling. The revised code may look like this:
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
return "Error: Division by zero is not allowed"
Now, we can re-run the AI review to verify the changes.
Use Cases of Multi-Agent AI in Coding
How it helps is this:
1. Automated Bug Detection
GPT-4 gives detailed explanations and alternatives, while DeepSeek detects syntax, logic, and security issues.
2. Code Optimization
Consider this inefficient Python function:
def sum_numbers(nums):
total = 0
for num in nums:
total += num
return total
DeepSeek may recommend sum() instead of a loop, and GPT-4 may optimize it using NumPy for huge datasets:
import numpy as np
def sum_numbers(nums):
return np.sum(nums)
This results in faster execution for large lists.
3. AI-Assisted Refactoring
Scalability and maintainability need rework. Multi-agent artificial intelligence might provide modular, reusable capabilities from twisted codes.
Advantages of Multi-Agent AI Systems
DeepSeek + GPT-4 has various benefits, as per my tests:
1. More Accurate Feedback
Context-aware, optimized recommendations based on coding best standards replace generic answers.
2. Reduced Developer Workload
This method is like having a clever helper, which saves time that would have been spent reviewing code manually.
3. Improved Performance and Security
By integrating AI models, I get greater security insights and performance gains that a single AI could miss.
Challenges and Considerations
Like every AI system, there are challenges:
1. Conflicting AI Suggestions
DeepSeek and GPT-4 could have different opinions. Manually examining both outputs and choosing the best insights solves this.
2. Computational Costs
Running numerous artificial intelligence models increases API costs and consumption. I suggest optimizing by looking at only significant code changes.
3. AI Understanding of Business Logic
While AI lacks business sense, it increases security and code structure. Human supervision is still very vital.
Conclusion
Our development process changed when we included multi-agent AI. Debugging, optimization, and code quality increase using DeepSeek analysis with GPT-4.
Use this multi-agent approach to improve and automated code review. It presents the direction of artificial intelligence-assisted development, in which AI makes informed contributions.
Want to incorporate multi-agent AI into your workflow? Let's discuss this in the comments!
139 views