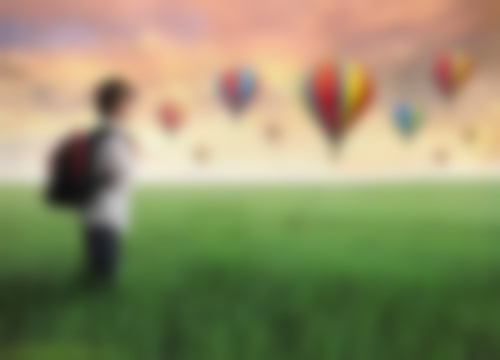
May 02, 2025
Implementing Gaussian Blur in C++ Using OpenCV
Have you thought about how Photoshop or real-time video processing blur images? Gaussian Blur is important to that smooth blur effect. Noise reduction and smoothing are common in image processing, and it is simple! I will show you Gaussian Blur in C++ using OpenCV, one of the most advanced image processing packages. Let us explore image processing and discover how to produce a professional blur effect with a few lines of code.
What is Gaussian Blur?
I will describe Gaussian Blur before writing the code. Gaussian Blur decreases noise and detail by averaging pixels in a neighborhood using a Gaussian function. It means that pixels closer to the blur center weigh more than ones farther away. The result? An attractive blur that softens edges and decreases detail.
Gaussian blur reduces image noise, creates bokeh, and detects edges when paired with other algorithms. It is an important image processing tool.
Setting Up the Environment
Install OpenCV to get started. No worries if you have not done this yet, I will help. Install OpenCV on your system first. You may install OpenCV using apt or brew or build it from source on Windows and Linux.
To install it, follow these simple steps:
- Windows users may get pre-built OpenCV libraries from the official website.
- For Linux/macOS, use package managers like sudo apt install libopencv-dev or brew install opencv.
- After installing, include the header files in your C++ project and start coding.
Code Walkthrough
Let us look at the code now. OpenCV makes image processing easy, therefore I will show you each step.
Step 1: Include OpenCV Header Files
Include OpenCV headers to use image processing functions:
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
Step 2: Load an Image
Please load your image to apply Gaussian Blur. Read the disk image using cv::imread()
cv::Mat image = cv::imread("path_to_your_image.jpg");
if (image.empty()) {
std::cout << "Could not open or find the image!" << std::endl;
return -1;
}
Step 3: Apply Gaussian Blur
Applying the blur is the primary element. OpenCV's cv::GaussianBlur() function accepts the source image, kernel size, and Gaussian kernel standard deviation. How to utilize it:
cv::Mat blurredImage;
cv::GaussianBlur(image, blurredImage, cv::Size(15, 15), 0);
In this example:
- cv::Size(15, 15) regulates blur size by specifying kernel size. Larger values blur more.
- In OpenCV, 0 indicates automated standard deviation calculation based on kernel size.
Step 4: Display the Result
Use cv::imshow() to view the original and blurred images:
cv::imshow("Original Image", image);
cv::imshow("Blurred Image", blurredImage);
cv::waitKey(0); // Wait for a key press
Step 5: Save the Blurred Image (optional)
If you'd like to save the blurred image, simply use cv::imwrite():
cv::imwrite("blurred_image.jpg", blurredImage);
Fine-Tuning the Parameters
After completing the basic implementation, let us fine-tune the parameters. Adjusting the kernel size and standard deviation are important. Smaller kernel sizes provide a more subtle blur, whereas bigger ones create a deeper blur.
Try these parameters to get the blur you want. Try values like cv::Size(5) for a modest blur, cv::Size(25, 25) for a stronger blur. To change blur strength, manually adjust the standard deviation (0 in the example).
Conclusion
This article showed how to make Gaussian Blur in C++ using OpenCV, a basic yet effective image processing tool. The code is simple, and you can adjust blur intensity by changing kernel size and standard deviation. Gaussian Blur is useful for noise reduction, background blurring, and other image modification tasks. Grab your images and try various parameters to find the perfect fit for your project!
262 views