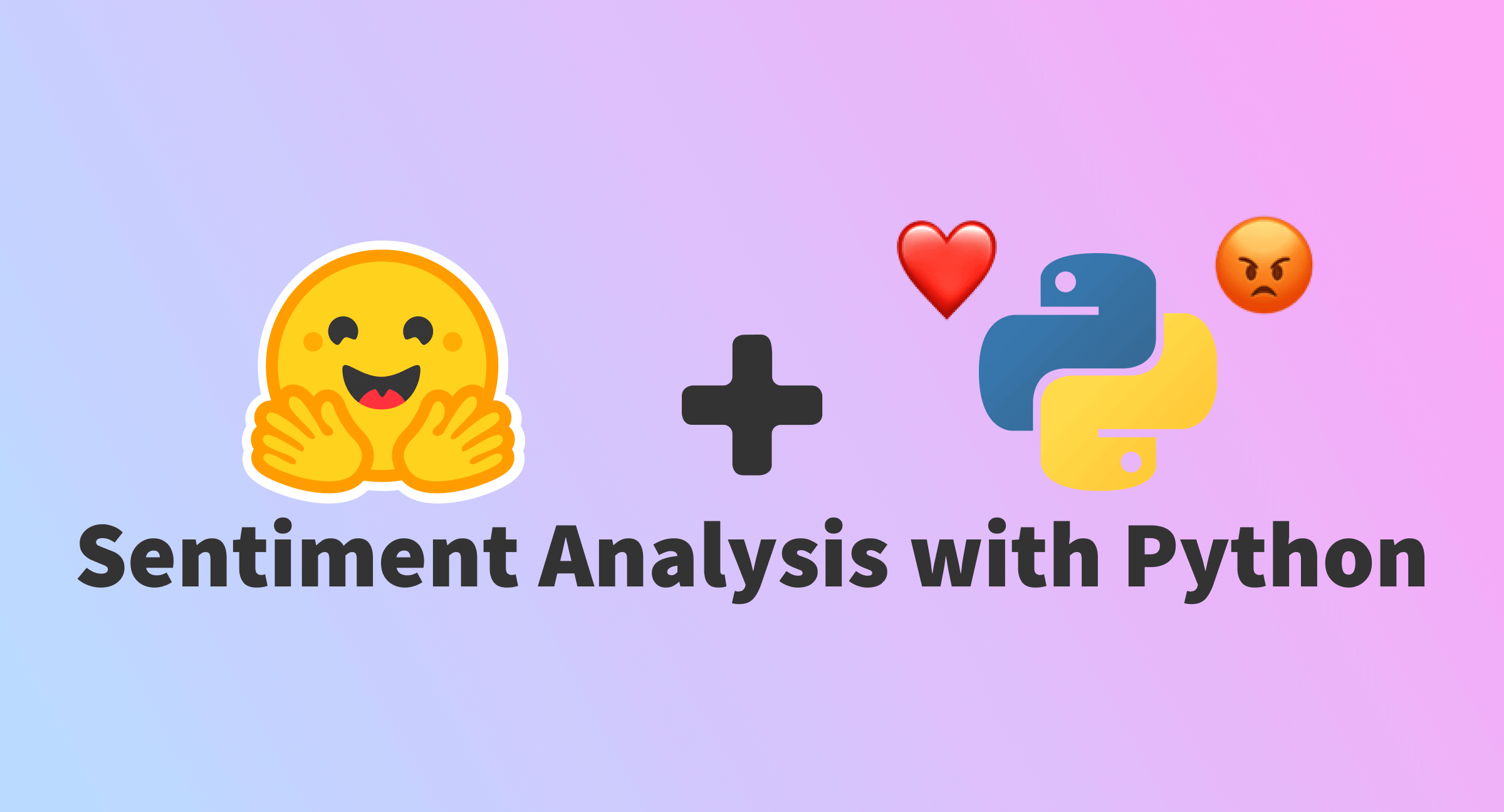
May 01, 2025
Python Sentiment Detector: A GUI Tutorial with Tkinter
Imagine being able to tell the tone of a text, an angry tweet or a nice product review, just from a few words. Cool, huh? Python and Tkinter make sentiment detectors possible. In this article, I will teach you how to develop a basic GUI-based sentiment analysis tool that can identify positive, negative, or neutral text. Python and ingenuity are enough, no data science degree required here!
Setting Up the Environment
Set up our environment before writing code. This project requires Tkinter and TextBlob (you might use VADER for more complex sentiment analysis, but we will use TextBlob for simplicity).
Install the essential libraries first. Launch your terminal and type:
pip install tk textblob
Now that everything is ready, let's briefly review these libraries' duties such as
- We utilize Tkinter to create a graphical user interface (GUI) for text input and results.
- TextBlob is a powerful Python library for NLP applications like sentiment analysis.
After that, let us begin!
Creating the GUI Layout
User interaction with the sentiment detector begins with the graphical interface. We will use Tkinter to create a simple window with a text input, sentiment analysis button, and result label.
Here's a basic Tkinter layout:
from tkinter import *
from textblob import TextBlob
# Initialize the GUI window
root = Tk()
root.title("Sentiment Detector")
# Add text box and button
text_input = Text(root, height=5, width=40)
text_input.pack()
def analyze_sentiment():
text = text_input.get("1.0", "end-1c")
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
if sentiment > 0:
result_label.config(text="Sentiment: Positive", fg="green")
elif sentiment < 0:
result_label.config(text="Sentiment: Negative", fg="red")
else:
result_label.config(text="Sentiment: Neutral", fg="blue")
button = Button(root, text="Analyze", command=analyze_sentiment)
button.pack()
result_label = Label(root, text="Sentiment: ", font=('Arial', 14))
result_label.pack()
root.mainloop()
Three primary GUI elements exist in this code. Users enter text in the Text widget. A multi-line text box with flexible input is ideal for evaluating any phrase or paragraph. Button is the second important part. It calls the analyze_sentiment() method to analyze the text input when clicked. Finally, the Label displays sentiment analysis results. The label turns green for positive, red for negative, and blue for neutral. These parts form a basic, interactive sentiment analysis tool with an easy-to-use UI.
Implementing Sentiment Analysis Logic
Now comes sentiment analysis, the fun part! TextBlob analyzes text emotion. TextBlob rates each text's polarity. This score is -1 (negative) to 1 (positive), with 0 neutral.
This is how we use it:
When the score is larger than 0, the sentiment is positive, if it is less than 0, it is negative, and if it is equal to 0, it is neutral.
In analyze_sentiment(), we utilize TextBlob to analyze user-entered content. For real-time feedback, we change label color based on polarity score.
Testing the Application
Our sentiment detector is ready for testing! Try these examples:
- Positive sentiment: "I absolutely love this app!"
- Negative sentiment: "This is the worst service I've ever used."
- Neutral sentiment: "The weather is fine today."
The result label color changes. Red means negative, green is positive, and blue means neutral. Just that simple!
Improving the Sentiment Detector
- Advanced models: Using more complex methods, like libraries or machine learning, to make your sentiment detector more accurate.
- Language detection: You can add features like language detection so that your sentiment detector can automatically detect languages to make it scalable.
- UI enhancements: You can add typefaces, background colors, and animations to improve the interface.
Adding these features would strengthen and simplify your app.
Conclusion
You now have a sentiment detector that was made with Python and Tkinter. We covered how to configure the system, constructing a basic GUI, and using TextBlob for sentiment analysis. Although basic, this app is a good introduction to Python-based natural language processing and GUI development.
You can modify the code, add features, and try alternative inputs. I welcome your questions and suggestions, let's keep the discussion going!
50 views