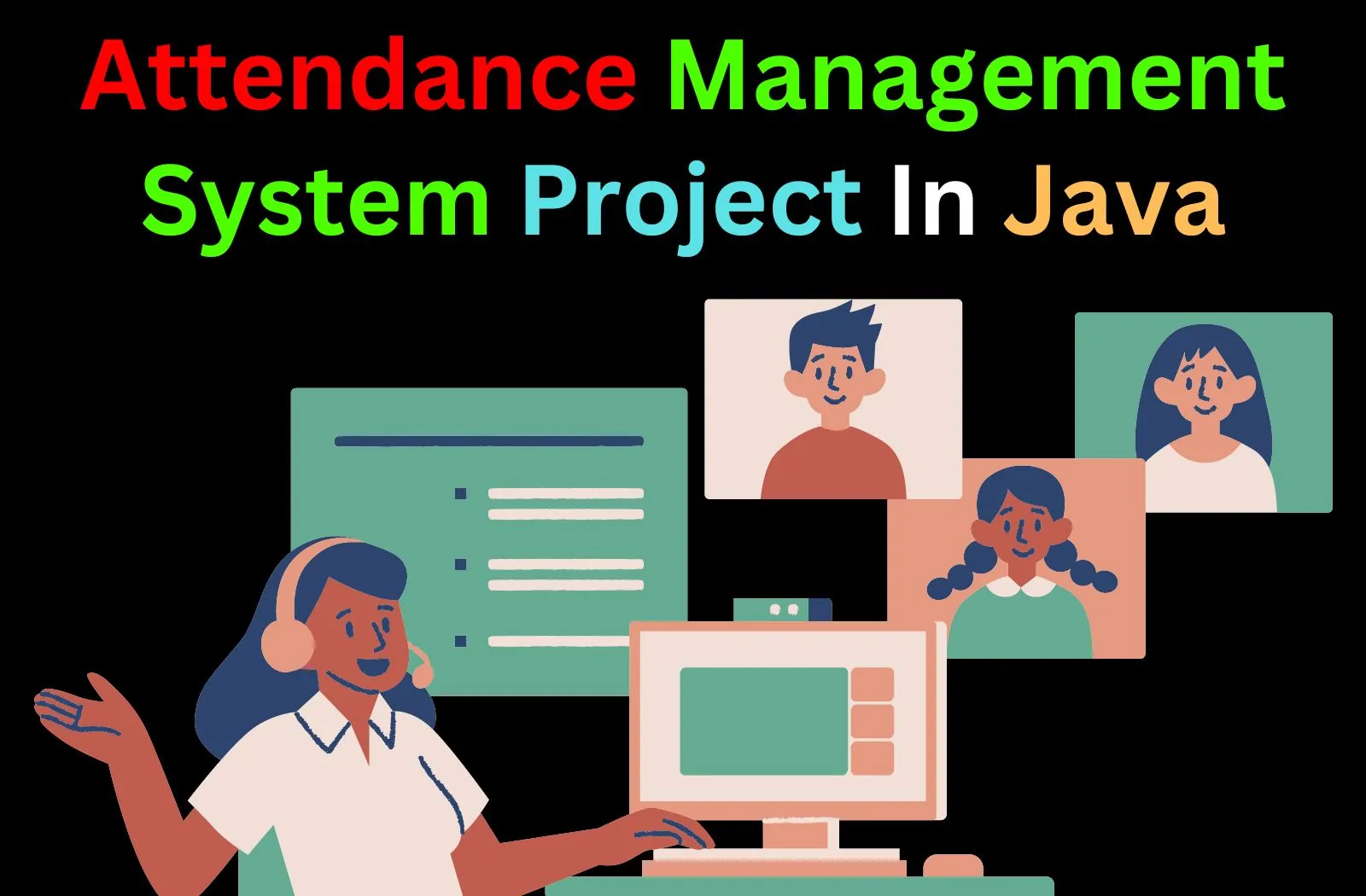
April 30, 2025
Create a Simple Java Attendance System
Have you ever struggled to manually keep track of class or work meeting attendance? A easier approach to automate the process? Start Java programming! A simple Java-based attendance system may reduce time and improve accuracy. This blog will show you how to use Java to maintain student or employee information, mark attendance, and create reports with a few lines of code. Go ahead and start!
Setting Up the Java Environment
Proper setup is necessary before coding. Install the Java Development Kit (JDK) to begin. Download it from Oracle's website. Select an IDE like IntelliJ IDEA, Eclipse, or NetBeans. These tools accelerate Java code writing and execution. After setting up your environment, you may write the automated attendance system program. Now let us design the system.
Designing the Attendance System
Understanding the attendance system's major components helps develop it. The system must store student or staff data, monitor attendance, and provide reports.
This basic system will keep each student's name, ID number, and attendance status. Use an ArrayList or HashMap to hold this data. We may link student names with attendance status in the HashMap from the ArrayList.
Additionally, we need these features too:
- Adding students: Facilitates new student data entry.
- Attendance recording: Recording student presence or absence.
- Generating a report: Reporting attendance status for each student at the ending of the session.
The system will satisfy key criteria with this structure. Next, code the main features.
Implementing the Core Features
Now, code the system's main features. First, create the Student class to store name and attendance.
public class Student {
String name;
boolean isPresent;
// Constructor
public Student(String name) {
this.name = name;
this.isPresent = false; // Default attendance is 'Absent'
}
// Method to mark attendance
public void markAttendance(boolean attendance) {
this.isPresent = attendance;
}
// Display attendance status
public void displayAttendance() {
String status = isPresent ? "Present" : "Absent";
System.out.println(name + ": " + status);
}
}
Next, we'll need a main class to manage students and mark attendance. Here's an example:
import java.util.ArrayList;
public class AttendanceSystem {
public static void main(String[] args) {
// Create a list of students
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("John"));
students.add(new Student("Sarah"));
students.add(new Student("Michael"));
// Mark attendance for each student
students.get(0).markAttendance(true); // John is Present
students.get(1).markAttendance(false); // Sarah is Absent
students.get(2).markAttendance(true); // Michael is Present
// Display the attendance report
for (Student student : students) {
student.displayAttendance();
}
}
}
This program lists students, records attendance, and shows status. The code is simple to modify for changes like adding students or updating attendance criteria.
Enhancing the System
Although the basic system works, it could be better. First, utilize JavaFX to construct a GUI for visual user interaction instead of console input. You may also use a database like MySQL or SQLite to store student information and attendance history when the application is closed. Adding export option to produce CSV or PDF attendance reports would also be helpful.
Such enhancements might make the attendance system more powerful.
Conclusion
Simple Java attendance systems save time and prevent human mistakes when keeping track of student or staff attendance. This blogpost shows you how to build a custom system and grow it as you learn Java programming.
521 views