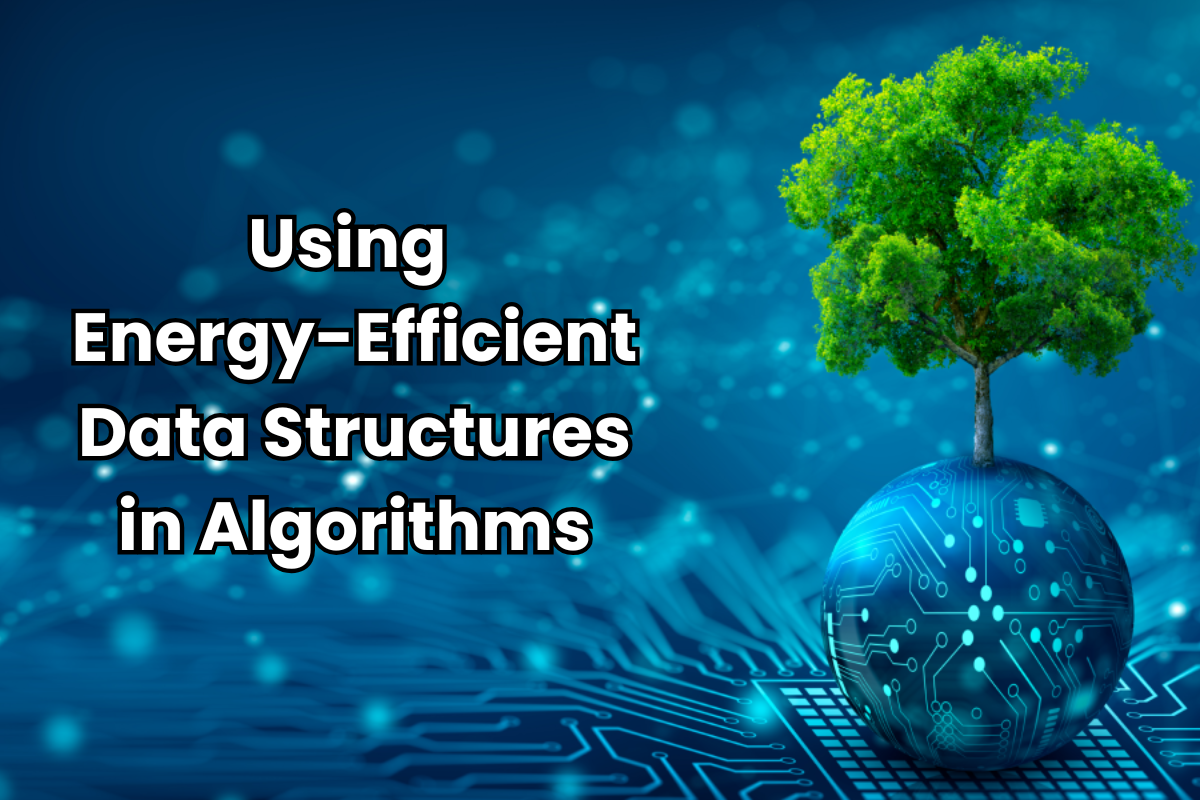
March 24, 2025
Using Energy-Efficient Data Structures in Algorithms
Do you know how much energy your code uses? In this era of climate consciousness and green technology, even tiny algorithm tweaks can save energy use. Data structure selection is a simple way to do this. This blog post will discuss how data structures effect energy efficiency and present Python examples for greener algorithms.
Understanding Energy Efficiency in Algorithms
Energy efficiency in computing reduces code execution power. These improvements may appear minor for small apps, but expanding to millions of users or enormous databases shows their importance.
Data structures allocate, access, and free memory. Choosing poor data structures may increase memory and processing costs, increasing energy use. Understanding this relationship lets us create eco-friendly, efficient algorithms.
Key Factors for Choosing Energy-Efficient Data Structures
- Memory Overhead: Efficient memory usage saves energy. The array module consumes less memory than Python lists for large datasets.
- Access Patterns: Lists take longer to search than sets, particularly for huge datasets, wasting energy.
- Algorithmic Complexity: The complexity of time impacts the length of the code. An O(1) lookup dictionary consumes less power than an O(n) list when working with big datasets.
Energy-Efficient Data Structures: Examples and Use Cases
Arrays and Lists:
Lists are larger than arrays, although arrays are superior for fixed-size collection operations.
import array
# Efficient for numerical operations
numbers = array.array('i', [1, 2, 3, 4])
print(numbers)
Use arrays when you do not have a lot of space and all the data types are the same.
Dictionaries and Hash Maps:
With O(1) complexity, dictionaries are great for quick lookups.
data = {"apple": 5, "banana": 3, "cherry": 7}
print(data["banana"]) # Fast and energy-efficient
You should use dictionaries to store key-value pairs that you need to look up often.
Heaps and Priority Queues:
Heaps are the best way to handle changing data with goals, like when you are scheduling tasks.
import heapq
tasks = []
heapq.heappush(tasks, (2, 'write blog'))
heapq.heappush(tasks, (1, 'review code'))
print(heapq.heappop(tasks)) # Most urgent task
You can easily manage sorted or prioritized data with them.
Sets:
Sets are great for quickly testing membership and getting rid of duplicates.
items = [1, 2, 3, 3, 4]
unique_items = set(items)
print(unique_items) # Removes duplicates
If you want to save time and energy when working with unique features, choose sets.
Trees:
Like binary search trees, trees are good at keeping data in order.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
Best Practices for Energy-Efficient Algorithm Design
These techniques have helped me create eco-friendly apps:
- Profile your code: Find code sections that are not working well by using tools like cProfile.
- Match Data Structure to Problem: Make sure that the data structures you choose fit the needs of the problem.
- Keep It Simple: Do not make things too complicated; smaller numbers might not need complex arrangements.
Conclusion
It may seem like a small step to improve energy efficiency, but it is an important one on the way to greener technology. If you pick the right data structures, you can use fewer resources while keeping or even better speed. You can try the above examples. Let's make code a little greener for the environment, one method at a time!
216 views