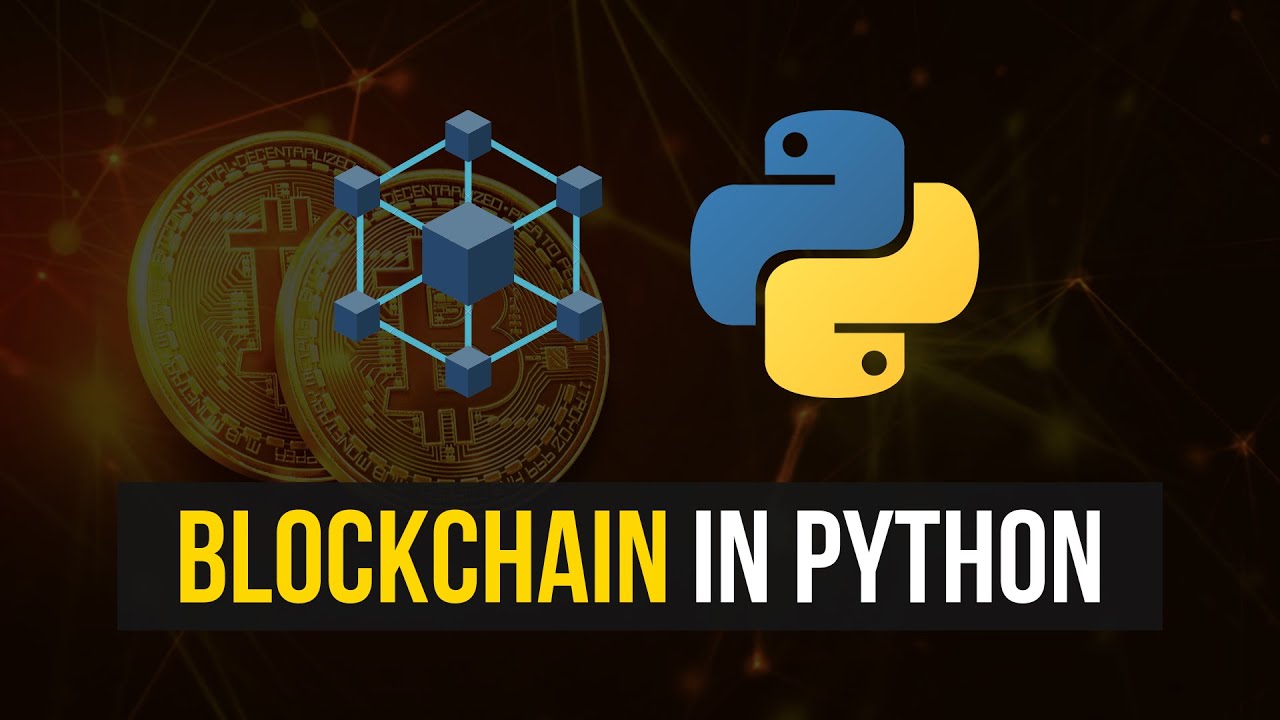
Have you ever thought about how trust works without a single authority in cryptocurrencies like Bitcoin? Blockchain technology is the answer. It is a novel idea that protects data by creating autonomous, unchangeable chains of data. You will learn how to make a simple blockchain in Python by following this step-by-step guide. There are steps you need to take to add blocks, hash data, and connect them to make your own secure chain. By the end, you will know how blockchains work and have a model that you can use to build on.
Prerequisites
Here's what you need before you start:
- A basic understanding of how to code in Python.
- Make sure Python is installed on your system.
- Know how to use the standard tools hashlib (for hashing) and datetime (for timestamps) in Python.
Let's get started!
Defining the Block Class
There are many blocks that make up a blockchain, and each one has important information in it. Each block has data, which is the information you want to keep, and a timestamp, which shows the exact time the block was made. Each block also has a previous hash that points to the hash of the block before it. This hash ensures that all blocks are safely linked in order. And, hash the block's contents, timestamp, and previous hash if you want to create a new hash. This unique identification keeps the blockchain stable and unchangeable.
Let's create a Block class:
import hashlib
import datetime
class Block:
def __init__(self, data, previous_hash):
self.timestamp = datetime.datetime.now()
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
hash_string = f"{self.timestamp}{self.data}{self.previous_hash}"
return hashlib.sha256(hash_string.encode()).hexdigest()
Calculate_hash mixes the timestamp, data, and preceding block hash to build a sha256 hash.
Creating the Blockchain Class
Since we have a Block class, let us create a Blockchain class to manage the chain. A genesis block will start the blockchain. This is the first block in the chain.
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block("Genesis Block", "0")
def add_block(self, data):
previous_block = self.chain[-1]
new_block = Block(data, previous_block.hash)
self.chain.append(new_block)
The create_genesis_block method creates a unique initial block for the blockchain. Since it starts the chain, the genesis block has no earlier number. Use add_block to add blocks to blockchain. Keep the chain safe by referencing the previous block's hash. Links between data blocks make them immutable and easy to verify.
Displaying the Blockchain
Let's implement a way to reveal block details to verify our blockchain:
def display_chain(self):
for block in self.chain:
print(f"Timestamp: {block.timestamp}")
print(f"Data: {block.data}")
print(f"Previous Hash: {block.previous_hash}")
print(f"Hash: {block.hash}\n")
We can see the whole chain because this method shows the date, data, previous hash, and current hash for each block.
Testing the Blockchain
Let's put our blockchain to the test! We will start a new blockchain, add a few blocks, and then show the chain to see what happens.
my_blockchain = Blockchain()
my_blockchain.add_block("Block 1: First transaction")
my_blockchain.add_block("Block 2: Second transaction")
my_blockchain.display_chain()
Sample Output:
Timestamp: 2024-04-10 12:34:56.789123
Data: Genesis Block
Previous Hash: 0
Hash: a3f1b2c5d6e8f9a0b1c2d3e4f5a6b7c8d9e0f1a2b3c4d5e6f7a8b9c0d1e2f3g4
Timestamp: 2024-04-10 12:35:00.123456
Data: Block 1: First transaction
Previous Hash: a3f1b2c5d6e8f9a0b1c2d3e4f5a6b7c8d9e0f1a2b3c4d5e6f7a8b9c0d1e2f3g4
Hash: b7f1d2a3c5e6f7g8a9b0c1d2e3f4g5h6a7b8c9d0e1f2g3h4i5j6k7l8m9n0o1p2q3
Timestamp: 2024-04-10 12:35:05.567890
Data: Block 2: Second transaction
Previous Hash: b7f1d2a3c5e6f7g8a9b0c1d2e3f4g5h6a7b8c9d0e1f2g3h4i5j6k7l8m9n0o1p2q3
Hash: c9e2f3d4a5b6c7d8e9f0a1b2c3d4e5f6a7b8c9d0e1f2g3h4i5j6k7l8m9n0o1p2q3r4
Conclusion
Congratulations! You created a basic Python blockchain. Blockchain basics include creating blocks, linking them, and verifying the chain. Here, you may add proof-of-work, mining payouts, and a consensus mechanism to your blockchain.
Blockchain technology is complicated, but breaking it down makes it easier. Experiment and you may design the next major decentralized app!
143 views