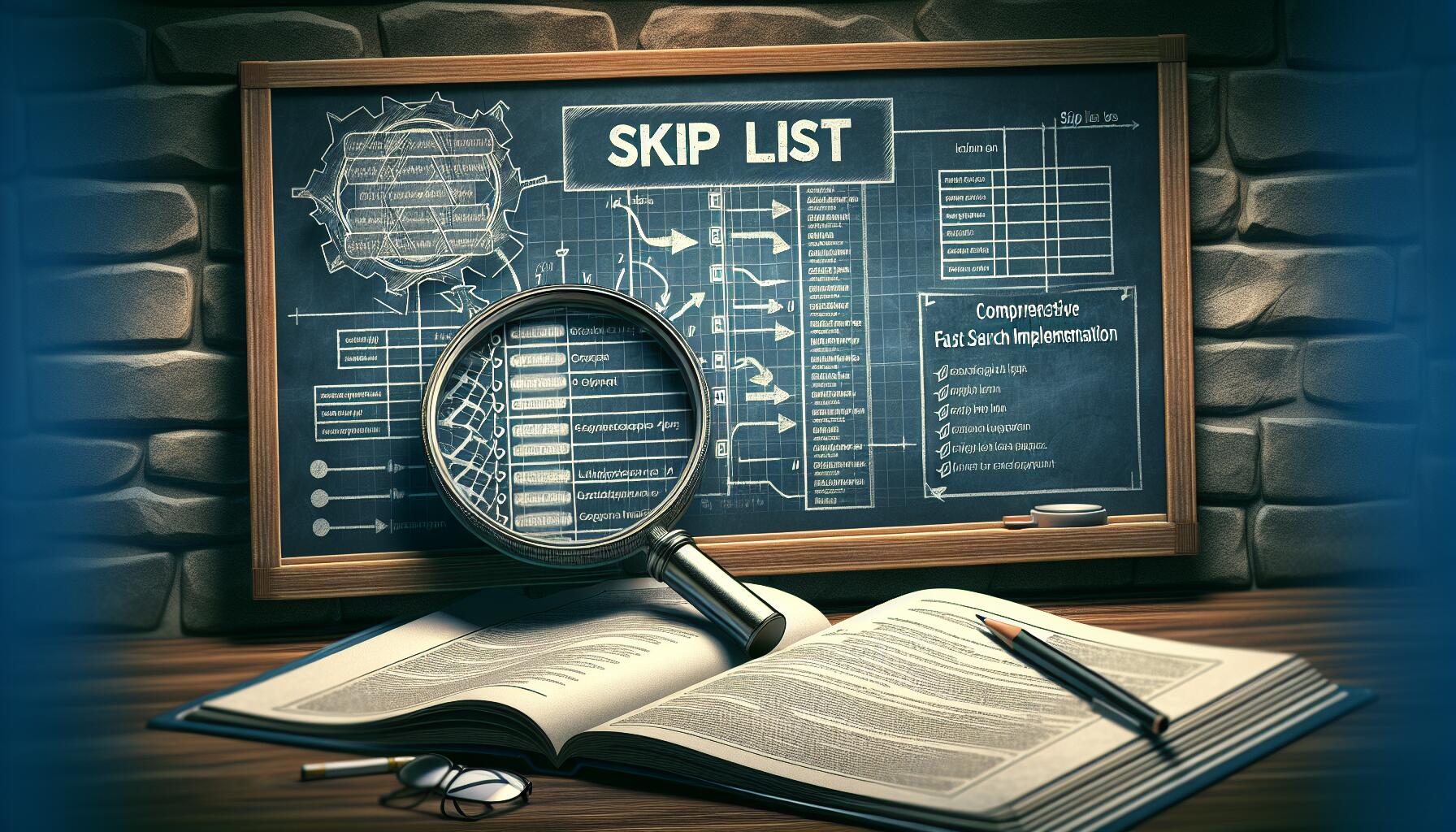
February 26, 2025
Implementing a Skip List for Fast Searching: A Modern Alternative to Linked Lists
Tired of sluggish linked list searches? Imagine a data structure that combines linked lists with binary search. Skip lists excel here! By "skipping" several nodes, skip lists efficiently store and search data. They are a contemporary alternative to linked lists that search quicker and are simpler.
This blog post explains skip lists, why they are valuable, and how to create one in Python.
Why Use Skip Lists Over Linked Lists?
Sequential data storage is wonderful with linked lists, but searching is poor. For bigger datasets, linked lists are inefficient because finding a particular item requires O(n) time.
Multiple link layers solve this problem in skip lists. These extra links let you skip portions of the list, minimizing comparisons. The result? A binary search tree-like average search time of O(log n). Skip lists are ideal for rapid lookups.
Structure of a Skip List
Layered skip lists are data highways. To speed up list navigation, add links (or levels).
- Nodes: Each skip list node has a value and several "forward links" to other nodes.
- Levels: The list contains numerous levels. Higher levels skip more nodes than the bottom linked list. Higher levels have fewer nodes.
If you are looking for a number in a skip list, you may skip big parts and descend down to reach the node.
How Skip Lists Work: Step-by-Step
Skip lists support search, insert, and delete. A simplified breakdown is below:
1. Search
- Start from the top.
- Move forward till you miss.
- Search the next level below.
- Repeat to finish or meet the goal.
2. Insertion
- Randomly choose the new node's levels.
- Find the best node placement at the highest level.
- Adjust pointers for the new node.
3. Deletion
- Search each level for its node.
- Update pointers to delete node references.
Python Implementation of a Skip List
A simple Python skip list implementation is below. Create Node and SkipList classes.
import random
class Node:
def __init__(self, value, level):
self.value = value
self.forward = [None] * (level + 1)
class SkipList:
MAX_LEVEL = 4
def __init__(self):
self.header = Node(None, self.MAX_LEVEL)
self.level = 0
def random_level(self):
level = 0
while random.random() < 0.5 and level < self.MAX_LEVEL:
level += 1
return level
def insert(self, value):
update = [None] * (self.MAX_LEVEL + 1)
current = self.header
for i in reversed(range(self.level + 1)):
while current.forward[i] and current.forward[i].value < value:
current = current.forward[i]
update[i] = current
level = self.random_level()
if level > self.level:
self.level = level
new_node = Node(value, level)
for i in range(level + 1):
new_node.forward[i] = update[i].forward[i]
update[i].forward[i] = new_node
# Example Usage
sl = SkipList()
sl.insert(5)
sl.insert(10)
sl.insert(7)
The Node Class represents each node with a value and forward pointers. The SkipList Class manages and inserts skips. The random_level method determines a node's level count. insert Method adds node and updates pointers.
Inserting items and adding search and remove functions are possible with this approach.
Benefits and Drawbacks of Skip Lists
Benefits:
- Skip lists search faster than linked lists, an average of O(log n).
- They are easier to install than AVL or Red-Black balanced trees.
Drawbacks:
- Multiple pointers per node increase skip list memory.
- Because skip lists are random, they behave inconsistently.
Conclusion
Skip lists are an innovative, fast search alternative to linked lists. They speed up search efficiency while simplifying design and execution by adding skip levels. Skip lists are a useful programming tool for datasets that demand frequent lookups or complicated data structures.
Implement a skip list in Python to see performance gains!
257 views