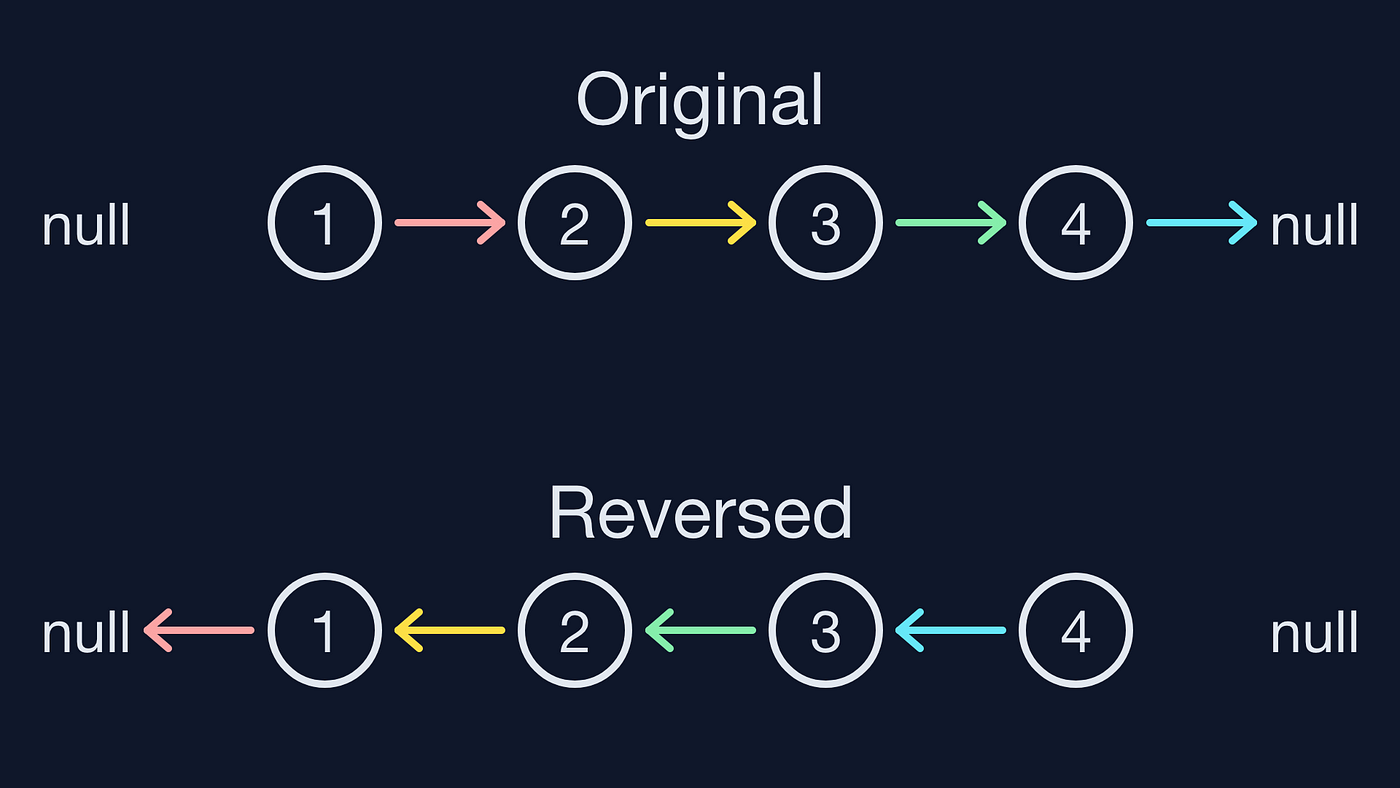
February 24, 2025
Reversing a Linked List: Iterative and Recursive Approaches in Java
Why is reversing a linked list a common coding interview question? It assesses data structures, algorithms, and code. Simple linked lists provide wonderful programming challenges. One problem is reversing linked list nodes. This blogpost will cover iterative and recursive Java techniques to solve this issue. This vital issue will be easy to solve thereafter!
What is a Linked List?
In a linked list, nodes hold items and point to the next node. Arrays store items in contiguous memory, whereas linked lists do not. Every node has two parts:
- Data: The value stored in the node.
- Next: A reference to the next node in the list.
For example:
1 -> 2 -> 3 -> null
The head node is 1, which points to 2, which points to 3, and null ends the list.
Reversing a Linked List: Problem Statement
The job is simple: Rank singly linked list nodes in reverse order. A list like this:
Input: 1 -> 2 -> 3 -> 4 -> null
We want to produce:
Result: 4 -> 3 -> 2 -> 1 -> null.
The key challenge is rewiring each node's next pointers while keeping list structure. Recursive and iterative approaches will fix this.
Iterative Approach
The iterative technique traverses the linked list and adjusts node next pointers. For this, we use prev, current, and next pointers.
How this approach works?
- Set prev to null and current to head.
- Loop until current is null.
- Save current.next in next for each loop.
- Reverse link via current.next to prev.
- Take prev and current one step forward.
- After the loop, prev becomes the new head of the inverted list.
Example:
class Node {
int data;
Node next;
Node(int data) { this.data = data; }
}
public Node reverseIterative(Node head) {
Node prev = null;
Node current = head;
while (current != null) {
Node next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
- Time Complexity: O(n) because we visit each node once.
- Space Complexity: O(1) due to few pointers.
Recursive Approach
Recursive approach solve subproblems to quickly reverse linked lists. Let's see its working below:
How this approach works?
- When head is null or head.next is null, return head.
- Head.next's function reverses the list.
- Revert by pointing the head.next.next to head.
- At last, set head.next to null to terminate the new list.
Example:
public Node reverseRecursive(Node head) {
if (head == null || head.next == null) return head;
Node newHead = reverseRecursive(head.next);
head.next.next = head;
head.next = null;
return newHead;
}
- Time Complexity: O(n) because we've visited each node once.
- Space Complexity: O(n) Due to the recursive call stack.
Conclusion
Every Java developer must learn to reverse a linked list. Practice these techniques to improve problem-solving and prepare for technical interviews. After understanding their workings and code examples, develop and execute these programs.
144 views