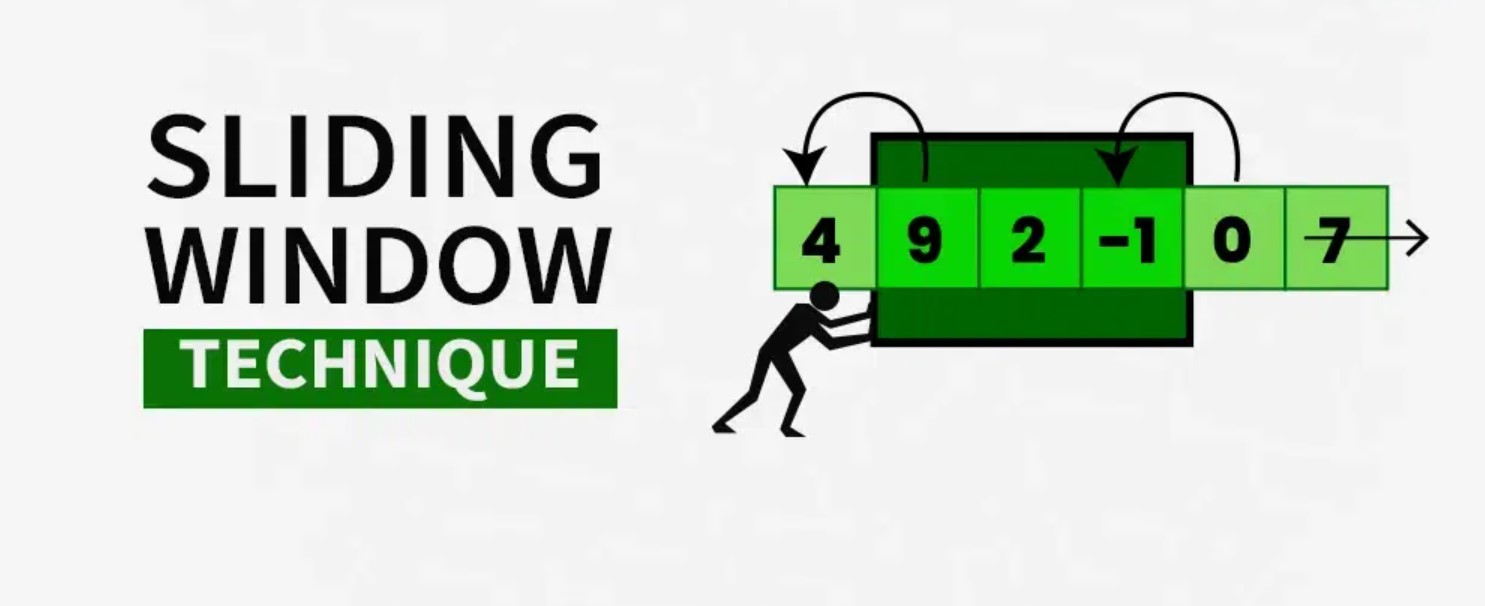
February 21, 2025
Sliding Window Technique: Optimizing Subarray Problems in Java and Python
Ever had a coding challenge when brute-force techniques are too slow? Efficiency matters when you're dealing with array and contiguous subarray issues. Find a subarray's highest sum or the longest substring without repeated characters. Trying all subarrays is very slow. The sliding window technique helps here. This technique improves efficiency by decreasing time complexity from O(n^2) to O(n). This blog post explains the sliding window concept and shows how to utilize it in Java and Python.
Types of Sliding Windows
The sliding window approach has two basic variations:
- Fixed Window Size: Keeps k-size constant. Computing values from the window's contents as you slide it. Example: Finding the largest sum of any k-sized contiguous subarray.
- Variable Window Size: Adjusts according to situations. This helps discover the longest substring without repeated characters.
Identifying the window size lets you apply the right reasoning and improve your solution.
Why Use the Sliding Window Technique?
When solving subarray or substring issues, brute-force techniques can take up to O(n^2) or more time by checking all potential subarrays. This is impractical for big data. Avoiding unnecessary computations with the sliding window approach reduces complexity to O(n).
Instead of recalculating the total for each new subarray, you "slide" the window of contiguous items by subtracting and adding. This technique is efficient, simple, and popular in competitive programming and data stream analysis.
Sliding Window in Action: Java and Python Examples
Example 1: Fixed-Size Sliding Window - Maximum Sum Subarray of Size K
Problem Statement: Find the maximum sum of each contiguous subarray of size k in an integer array.
Java Code:
public int maxSumSubarray(int[] arr, int k) {
int maxSum = 0, windowSum = 0;
// Calculate the sum of the first window
for (int i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window
for (int i = k; i < arr.length; i++) {
windowSum += arr[i] - arr[i - k];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
Python Code:
def max_sum_subarray(arr, k):
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum += arr[i] - arr[i - k]
max_sum = max(max_sum, window_sum)
return max_sum
Example 2: Variable-Size Sliding Window - Longest Non-Repeating Substring
Problem Statement: Find a string's longest non-repeated substring.
Java Code:
public int lengthOfLongestSubstring(String s) {
Set<Character> seen = new HashSet<>();
int left = 0, maxLength = 0;
for (int right = 0; right < s.length(); right++) {
while (seen.contains(s.charAt(right))) {
seen.remove(s.charAt(left));
left++;
}
seen.add(s.charAt(right));
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
Python Code:
def length_of_longest_substring(s):
seen = set()
left = 0
max_length = 0
for right in range(len(s)):
while s[right] in seen:
seen.remove(s[left])
left += 1
seen.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
Key Takeaways and Best Practices
- When to Use: Apply the sliding window method to continuous sequences in arrays or strings.
- Fixed vs. Variable Windows: Figure out if the size of your windows needs to stay the same or change dynamically.
- Efficiency Tip: Slide the window to update sums or conditions instead of recalculating values.
- Pitfalls: Avoid potential issues by carefully defining window limits and criteria for expansion or contraction.
Conclusion
Subarray and substring problems benefit from sliding window optimization. Avoiding brute-force speeds up huge dataset solutions. Programmers must learn this approach for dynamic substring and fixed-size sum computations.
Consider sliding windows for continuity. Use coding challenges to enhance productivity!
239 views