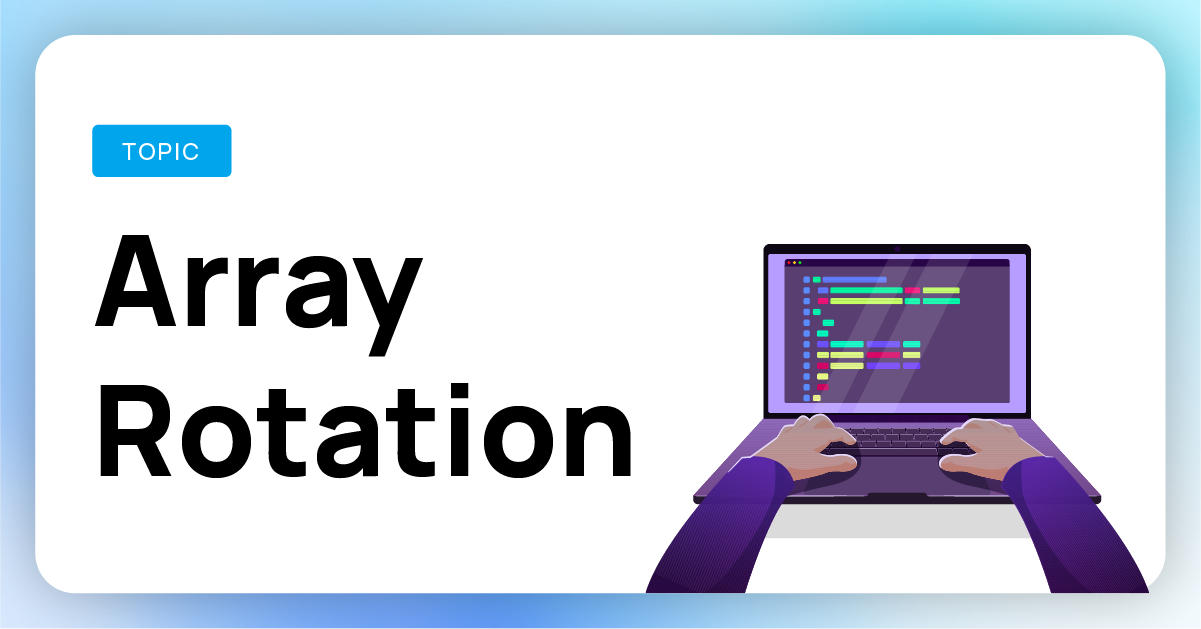
February 17, 2025
Efficiently Rotating Arrays: Implementations in Python, Java, and C++
Have you tried reordering array elements for data manipulation or competitive programming? Shifting elements left or right many times is a typical array rotation issue. Despite its simplicity, inefficient methods might slow down your program while handling massive datasets. I'll discuss left and right rotations, their complexities, and efficient Python, Java, and C++ implementations in this blogpost.
Understanding Array Rotations
What is a Left Rotation?
Left rotation moves each array element by kkk. The end reattaches starting elements.
Example:
For the array [1, 2, 3, 4, 5] rotated left by 2 positions, the result is [3, 4, 5, 1, 2].
What is a Right Rotation?
Right rotation moves each element kkk places. Reattachments are made at the start of moving elements.
Example:
For the array [1, 2, 3, 4, 5] rotated right by 2 positions, the result is [4, 5, 1, 2, 3].
Why Efficient Solutions Matter
Optimizing array rotations is essential in huge datasets or time-sensitive situations like coding contests. A basic technique can work for small inputs, but millions of elements need efficiency.
Naive Approach to Array Rotations
Using a loop to rotate elements one at a time is easiest. For each rotation, move each element one place.
Pseudo-code for Left Rotation:
for i from 1 to k:
temp = arr[0]
for j from 0 to n-2:
arr[j] = arr[j+1]
arr[n-1] = temp
Drawbacks:
The time complexity of this approach is O(n x k), where nnn is the array length and kkk is the rotation count. Large kkk numbers make this inefficient.
Efficient Approach for Array Rotations
Reversal is the best array rotation method. This approach takes O(n) time and O(1) space. To rotate left by kkk places:
- Reverse the first kkk elements.
- Reverse the remaining n-k elements.
- Reverse the array.
Right rotation has different split points but is comparable.
Implementation in Python
Left Rotation in Python
def left_rotate(arr, k):
n = len(arr)
k = k % n
arr[:] = arr[k:] + arr[:k]
# Example usage
arr = [1, 2, 3, 4, 5]
left_rotate(arr, 2)
print(arr) # Output: [3, 4, 5, 1, 2]
Right Rotation in Python
def right_rotate(arr, k):
n = len(arr)
k = k % n
arr[:] = arr[-k:] + arr[:-k]
# Example usage
arr = [1, 2, 3, 4, 5]
right_rotate(arr, 2)
print(arr) # Output: [4, 5, 1, 2, 3]
Left rotation uses arr[k:] + arr[:k] to slice and reorder the array. For right rotation, use arr[-k:] + arr[:-k] from the end.
Implementation in Java
Left Rotation in Java
public static void leftRotate(int[] arr, int k) {
int n = arr.length;
k = k % n;
reverse(arr, 0, k - 1);
reverse(arr, k, n - 1);
reverse(arr, 0, n - 1);
}
Right Rotation in Java
public static void rightRotate(int[] arr, int k) {
int n = arr.length;
k = k % n;
reverse(arr, 0, n - k - 1);
reverse(arr, n - k, n - 1);
reverse(arr, 0, n - 1);
}
Helper Function for Reversal
private static void reverse(int[] arr, int start, int end) {
while (start < end) {
int temp = arr[start];
arr[start++] = arr[end];
arr[end--] = temp;
}
}
Implementation in C++
Left Rotation in C++
#include <iostream>
#include <algorithm>
void leftRotate(int arr[], int n, int k) {
k = k % n;
std::rotate(arr, arr + k, arr + n);
}
Right Rotation in C++
void rightRotate(int arr[], int n, int k) {
k = k % n;
std::rotate(arr, arr + n - k, arr + n);
}
std::rotate from the C++ Standard Library rotates the array efficiently. The function rotates the range [arr, arr + k, arr + n] to achieve the desired rotation.
Comparing Time Complexities
- The Naive Approach O(n x k): is inefficient for big datasets.
- Efficient Approach: O(n): linear time, independent of kkk.
- Space complexity: O(1): no additional space in the efficient technique.
Conclusion
For programmers, efficiently rotating arrays is essential. For big datasets, efficient algorithms like the reversal algorithm are far better than naive solutions. To handle left and right rotations, I examined Python, Java, and C++ solutions. Now, look into and apply these solutions to your coding problems!
183 views