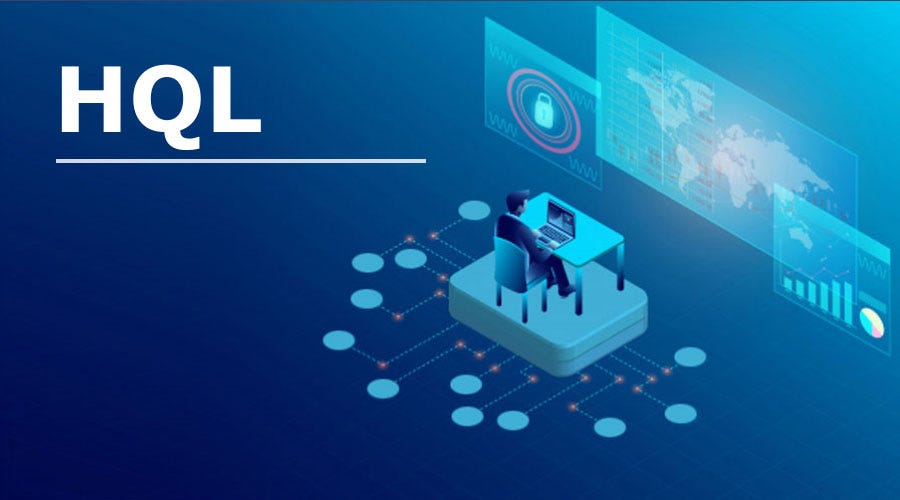
February 14, 2025
Fetching and Saving Data with Hibernate Query Language (HQL) in Java
Have you struggled with sophisticated SQL queries in Java applications? What if creating queries in a Java-specific language simplified database interactions? The elegant Hibernate Query Language (HQL) simplifies database processing. This article will demonstrate how HQL speeds up data retrieval and storage, making database interactions more intuitive and Java-like. Learn HQL for efficient Java data processing.
What is HQL?
Hibernate's HQL is a powerful query language. Java works with databases using this object-oriented query language. HQL uses Java classes and objects, not databases. Integrates Java code to relational databases effortlessly, making it excellent for Hibernate.
HQL beats SQL due to its object-oriented approach and Java class-based queries over database tables. HQL queries use FROM Student instead of SELECT * FROM Student. By abstracting the database schema and making your code more intelligible, HQL reduces your database implementation dependence.
Fetching Data with HQL
Data retrieval with HQL is simple yet powerful, allowing object queries. Its syntax resembles SQL but focuses on entities rather than tables.
Basic Fetching Example
Here's a sample Student class in a Java project. Basic HQL queries fetch all students from the database:
String hql = "FROM Student";
Query query = session.createQuery(hql);
List<Student> students = query.list();
Advanced Fetching Techniques
Use WHERE, ORDER BY, and GROUP BY clauses to filter, sort, and group findings.
- WHERE Clause: Fetching students with a specific condition (e.g., grade greater than 80).
String hql = "FROM Student WHERE grade > 80";
Query query = session.createQuery(hql);
List<Student> topStudents = query.list();
- ORDER BY Clause: Student names sorted ascendingly.
String hql = "FROM Student ORDER BY name ASC";
Query query = session.createQuery(hql);
List<Student> sortedStudents = query.list();
- GROUP BY Clause: Grouping students by their grade.
String hql = "SELECT grade, COUNT(*) FROM Student GROUP BY grade";
Query query = session.createQuery(hql);
List<Object[]> result = query.list();
Saving Data with HQL
HQL can save and update database data as well as fetches it. Hibernate's session object has different data-persistence methods.
Saving Data
To store a new Student record in the database using HQL, utilize the Session object's save() method:
Student student = new Student("John", 90);
session.save(student);
This creates a Student object and session.save(student) persists data to the database.
Updating Data
HQL enables updating existing records. You may update an entity using update():
String hql = "UPDATE Student SET grade = :newGrade WHERE id = :studentId";
Query query = session.createQuery(hql);
query.setParameter("newGrade", 95);
query.setParameter("studentId", 1);
query.executeUpdate();
We update the grade of a student with a specific id and query.setParameter() binds the parameter values to the query.
Using saveOrUpdate()
Hibernate's saveOrUpdate() function can add or update an entity depending on its identifier.
Student student = new Student("Emma", 88);
session.saveOrUpdate(student);
This approach is handy when you are not sure whether the entity is in the database.
Optimizing Data Operations
HQL simplifies data retrieval and storing, however database performance improvement is always important. Hibernate optimizes queries various ways:
- Pagination: Use pagination to access big quantities of data in segments instead than loading everything at once.
String hql = "FROM Student";
Query query = session.createQuery(hql);
query.setFirstResult(0);
query.setMaxResults(10);
List<Student> page1 = query.list();
- Caching: Caching speeds up data retrieval for frequently requested entities by reducing database queries in Hibernate.
Conclusion
Hibernate Query Language (HQL) provides complex and simple database interaction in Java applications. HQL simplifies data processes and enhances productivity using Java objects and SQL syntax. HQL lets Hibernate developers get data with simple queries and save and edit records. Use HQL in Java programs now to increase database operations and code efficiency.
214 views