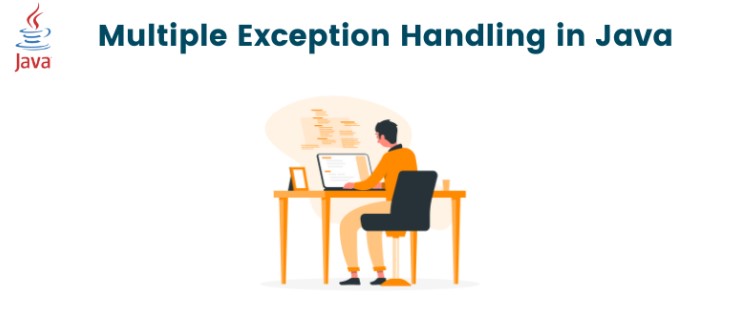
February 10, 2025
Handling Multiple Exceptions in Java: Best Practices with Examples
Have you tried handling multiple exceptions in one try block? You have undoubtedly seen code raise multiple exceptions in bigger Java programs. Java code often handles exceptions, but managing numerous exceptions effectively may improve readability, maintainability, and error-freeness. This article will provide best practices for handling many Java exceptions and examples to improve your error-handling process.
Handling Multiple Exceptions in a Single Try Block
Multiple catch blocks are a typical Java method for handling numerous exceptions. This method lets you handle exception types individually.
Example:
try {
// Some code that may throw different exceptions
FileInputStream file = new FileInputStream("file.txt");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/db", "user", "password");
} catch (IOException e) {
// Handle IOException
System.out.println("File error: " + e.getMessage());
} catch (SQLException e) {
// Handle SQLException
System.out.println("Database error: " + e.getMessage());
}
We've used separate catch blocks for IOException and SQLException in the given code. Although simple, this technique might cause duplication and a bloated codebase, particularly when multiple exceptions share handling logic.
Using Multi-Catch Syntax
Java 7 introduces multi-catch, which lets you catch many exceptions in one block. Handling exceptions the same manner simplifies code.
Example:
try {
// Some code that may throw different exceptions
FileInputStream file = new FileInputStream("file.txt");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/db", "user", "password");
} catch (IOException | SQLException e) {
// Handle both exceptions in a single block
System.out.println("Error: " + e.getMessage());
}
IOException and SQLException share a catch block. We can set up multiple exception types using | operator. When the exception handling logic is the identical for both exceptions, this method reduces repetition and simplifies the code.
Best Practices for Handling Multiple Exceptions
To keep your code clean and efficient while handling many exceptions, adopt these recommended practices.
- Catch only what you can handle: Avoid generic exceptions like Exception unless required. Identify and handle exceptions. This prevents you from missing additional mistakes.
- Order of catching exceptions: Catch specified exceptions first. Catching a generic exception first prevents the program from reaching the specialized ones, making them difficult to handle.
- Meaningful exception handling: To handle exceptions meaningfully, use catch blocks to report errors or provide helpful messages to the user. A general âerror occurredâ notice lacks context for debugging.
- Avoid overusing multi-catch: While it simplifies code, it may not be the best answer. When handling both exceptions is identical, use it. Use numerous catch blocks for handling various exceptions.
Advanced Scenario: Catching Multiple Exceptions and Logging
For troubleshooting and monitoring bigger apps/programs, particularly in production, logging exceptions can give significant insights. We've caught multiple exceptions for analysis.
Example:
try {
// Code that may throw different exceptions
FileInputStream file = new FileInputStream("file.txt");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/db", "user", "password");
} catch (IOException | SQLException e) {
// Log the exception for further analysis
logger.error("Exception caught: " + e.getMessage(), e);
}
We log the exception details and stack trace here to offer additional error information. This helps in production scenarios when real-time debugging is not feasible.
Conclusion
Java programming must handle exceptions well to be clean and maintainable. Multi-catch simplifies block exception handling without compromising readability in Java. This functionality lets you catch multiple exceptions, get appropriate error alerts, and use catch blocks wisely. Follow these recommended practices to make your code more resilient and debuggable.
531 views