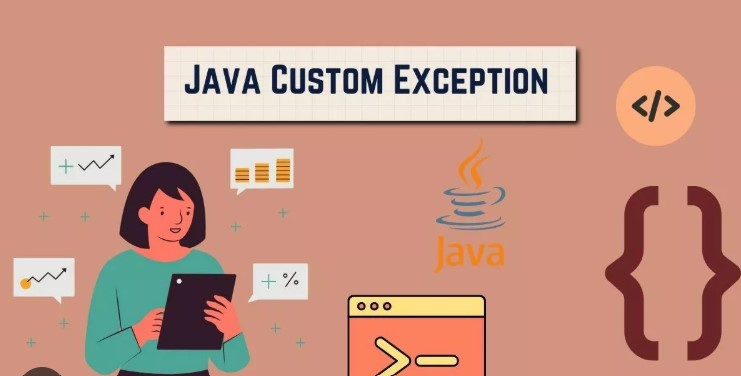
February 07, 2025
Custom Exceptions in Java: Creating and Using Your Own Error Classes
Has a generic error message ever disappointed you? If your program throws a vague exception like NullPointerException or ArrayIndexOutOfBoundsException, you have no idea what happened. Here come custom exceptions. In Java, developing your own error classes lets you create more explicit, informative, and meaningful exceptions to better handle code failures. Let's discuss how to build and utilize custom exceptions to improve Java apps.
Defining Custom Exceptions
Java custom exceptions are easy. A class that implements Exception or RuntimeException can handle checked or unchecked exceptions. Let's explore custom exception definition.
Here's an example of defining a checked exception:
public class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
InvalidAgeException extends Exception, making it checked. The constructor lets us send a custom error message to Exception.
To produce an unchecked exception, extend RuntimeException instead of Exception:
public class InvalidLoginException extends RuntimeException {
public InvalidLoginException(String message) {
super(message);
}
}
If appropriate, you can add properties or methods to your own exception class to hold error codes and context.
Throwing and Catching Custom Exceptions
Throw and catch your custom exception in your application after defining it. A try-catch block handles custom exceptions thrown using the throw keyword.
Example of throwing and catching a custom exception:
public class AgeValidator {
public static void validateAge(int age) throws InvalidAgeException {
if (age < 0) {
throw new InvalidAgeException("Age cannot be negative.");
}
}
public static void main(String[] args) {
try {
validateAge(-5);
} catch (InvalidAgeException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
This example throws InvalidAgeException if age is negative. The catch block prints the error message after catching the exception.
Best Practices for Using Custom Exceptions
Used wisely, custom exceptions are powerful. Here are several Java application custom exception recommended practices:
- Meaningful names: Name your own exception classes descriptively. MyException is very unclear, but InvalidAgeException clearly states the mistake.
- Avoid overuse: Use custom exceptions when current ones lack clarity or information to avoid misuse. Avoid generating custom exceptions for every error. Use as required to avoid complexity.
- Provide useful messages: Custom exceptions should help developers find errors. Error codes and context may assist.
- Catch at appropriate levels: Catch application exceptions at the correct level. For example, catching custom exceptions early in the code can conceal the problem's origin.
Real-World Use Cases of Custom Exceptions
Custom exceptions can enhance many real-world applications. Custom exceptions are useful in several situations:
- User input validation: Handle specific errors with custom exceptions like InvalidAgeException or InvalidEmailFormatException in online forms or registration systems.
- Business logic errors: Custom exceptions, such as PaymentFailedException or InsufficientFundsException, help clarify business logic problems in payment processing systems.
- Database connection issues: Add exceptions like DatabaseConnectionException to address particular database connection problems instead to general ones.
Custom exceptions let developers rapidly find and repair issues, resulting in cleaner, more maintainable code.
Conclusion
Java custom exceptions help write clear, maintainable, and informative error handling code. Your applications will benefit from clear, thorough error alerts and better debugging and problem-solving. Make Java programs more stable and maintainable with these tips. Create custom exceptions in your next project to enhance your code and debugging if you are new to exception handling.
429 views