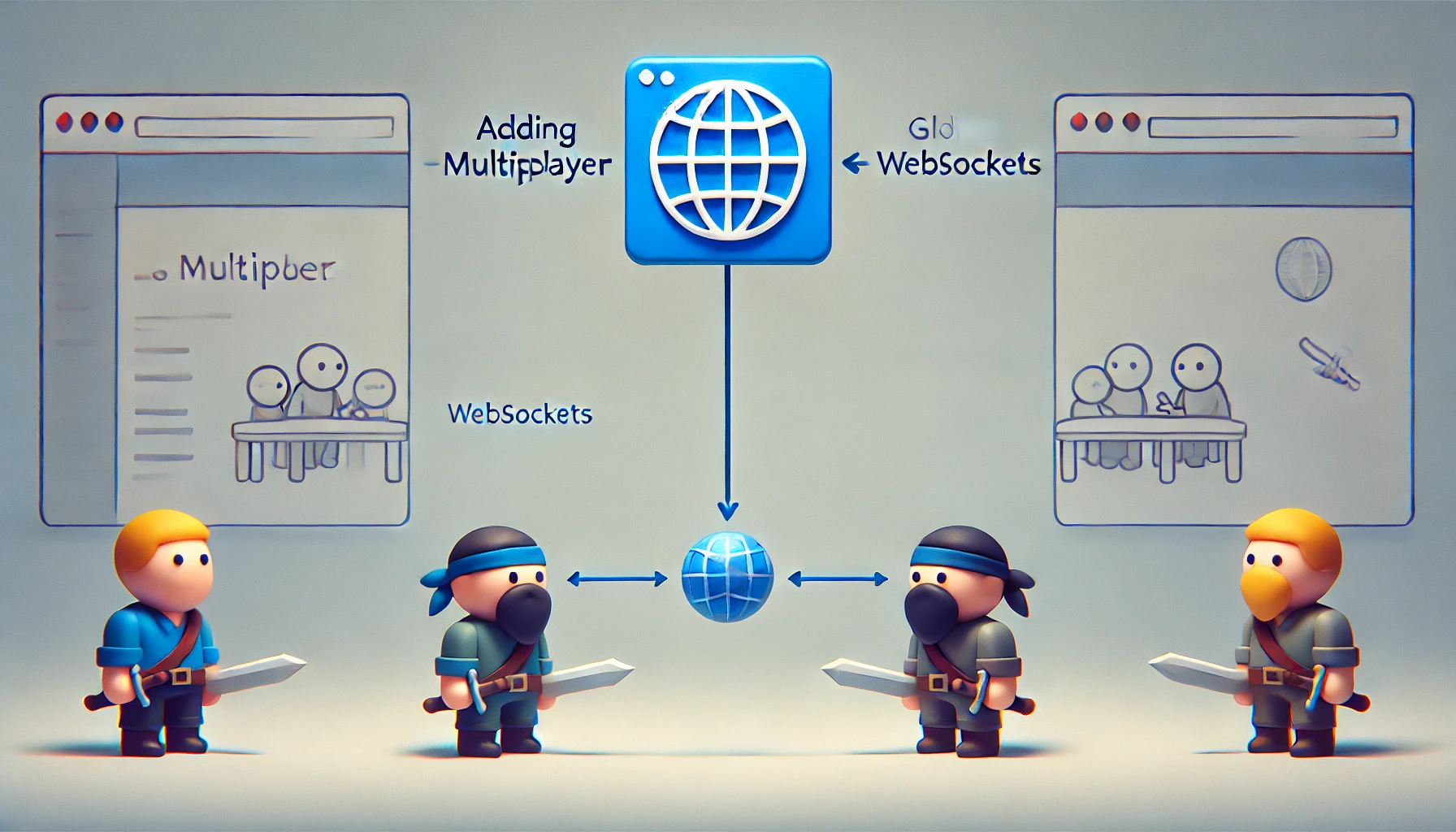
January 15, 2025
Adding Multiplayer to Your 2D Game with WebSockets
Want to bring your 2D game to life with real-time player interaction? WebSockets provide quick, persistent server-player communication, making this fantasy possible. This post explains how to implement WebSockets multiplayer capability.
Why WebSockets for Multiplayer Games?
Traditional HTTP queries are not suitable multiplayer games. Slow and inefficient for continuous, two-way communication. Here come WebSockets. WebSockets allow servers and clients to transmit and receive messages immediately without needing to reconnect. Multiplayer games need real-time updates, thus this low-latency connectivity is ideal. WebSockets let you communicate player actions, synchronize game states, and give live event-driven interactions, making your 2D game dynamic and interesting.
Setting Up the Server
We need a WebSocket server to connect numerous players. Setting this up using Node.js and the ws library is simple. Let's discuss a simple WebSocket server that can help you handle connections and messages:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
});
ws.send('Hello from server!');
});
This server accepts WebSocket connections on port 8080. It records client messages. New clients get a welcome message. Manage several players and send messages to all connected clients from here.
Creating the Client
Let us develop the server-interacting client. The method is same for frameworks like Phaser and simple JavaScript. Create a JavaScript WebSocket client:
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = () => {
console.log('Connected to server');
socket.send('Player connected');
};
socket.onmessage = (event) => {
console.log('Message from server:', event.data);
// Update game state based on server message
};
After the onopen event, the client sends a message that tells the WebSocket server about the player's link. After the server delivers data (e.g., game state changes), the game updates using the onmessage event. With this connection, you can transmit player actions (e.g., movement, attacks) to the server and get real-time player updates.
Synchronizing Game State
Multiplayer games must synchronize game state across all participants. In 2D games, it is possible to sync data like player places, health, scores, and more. The server should let all players know what is going on with the game. Delta updates save energy by only sending the changes to clients. And the server tells all clients about new player positions:
// Example: server sends updated player position to all clients
wss.clients.forEach((client) => {
client.send(JSON.stringify({ type: 'update_position', x: player.x, y: player.y }));
});
Testing and Optimization
Implementing multiplayer requires testing. For multiple clients You should test for latency, synchronization, and performance. Monitoring WebSockets and browser developer tools can optimize message size and identify bottlenecks. Moreover, it is important to keep server load and disconnections under control.
Conclusion
So, here our article ends and hope you get all the understanding of the content. WebSockets allow a 2D game to include multiplayer capabilities with real-time communication and minimal latency. So, set up a WebSocket server and connect clients to make your game multiplayer. Moreover, you can add real-time chat or leaderboards to improve your game!
905 views