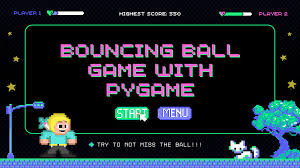
January 13, 2025
Physics-Based Gameplay: Creating a Bouncing Ball Game from Scratch
How can developers make games using real-world objects? Physics-based gaming, where mathematical models imitate real-world physics, makes games more immersive. We will make a simple, enjoyable physics-based bouncing ball game today. Let's start from scratch and see how coding can move the ball!
Understanding the Basics of Physics in Games
Video game physics goes beyond mathematics. Gameplay should feel authentic and participatory. Gravity, velocity, acceleration, and friction help us do this. These aspects determine game objects' movement and interaction. Gravity, velocity, and how the ball bounces will dominate our bouncing ball game. Understanding these fundamentals will help you create realistic games.
Setting Up the Development Environment
We need to make sure everything works before we start writing code. We will use Pygame, a well-known Python 2D game tool, because it is easy to use. To start installing Pygame, run the code below:
pip install pygame
The Code Behind the Ball's Movement
Now its time to code the ball's movement. Simple Pygame bouncing ball implementation. A bounce factor and gravity will cause the ball to descend.
import pygame
import sys
# Initialize Pygame
pygame.init()
# Screen setup
screen = pygame.display.set_mode((800, 600))
clock = pygame.time.Clock()
# Ball properties
ball_pos = [400, 300]
ball_velocity = [0, 0]
gravity = 0.5
bounce_factor = -0.7
# Main game loop
while True:
screen.fill((0, 0, 0)) # Clear screen
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Physics calculations
ball_velocity[1] += gravity # Apply gravity
ball_pos[1] += ball_velocity[1] # Update position
# Ball collision with ground
if ball_pos[1] >= 600 - 20: # Ball reaches bottom of screen
ball_pos[1] = 600 - 20
ball_velocity[1] *= bounce_factor # Bounce effect
pygame.draw.circle(screen, (255, 0, 0), (int(ball_pos[0]), int(ball_pos[1])), 20)
pygame.display.flip()
clock.tick(60) # 60 FPS
Enhancing the Game with Advanced Features
Once the base game works, let us make it better. You can change the ball's height by changing its gravity and bounce factor. Want a game that is more fun? Add wall collision! To get the ball to bounce off the screen, slow it down horizontally as it gets closer to the x-position.
User input is another choice. Let the player click on the screen to pick how fast the ball moves. This will make the game more fun.
Conclusion
A few lines of code are all it takes to make our simple physics-based bouncing ball game work! To simulate ball movement and bounce, we developed our programming environment, using basic physics, and wrote code. But this is just the beginning! It might be more fun to change the settings and add user input or hurdles. So explore with your own ideas and level up this bouncing ball!
479 views