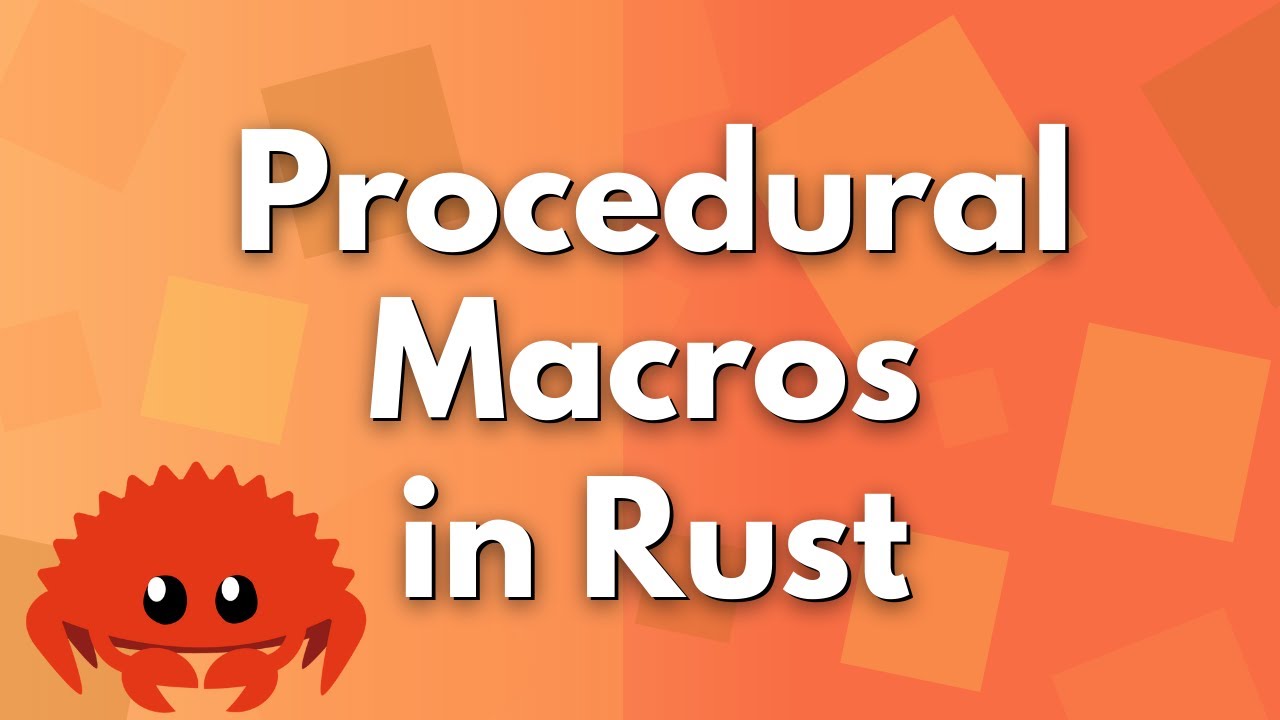
January 10, 2025
Rust Macros: Metaprogramming with Procedural Macros
Want your code to write itself? Macros in Rust produce code at compile time, minimizing boilerplate and repeated chores. However, procedural macros stand out. Complex metaprogramming with these strong tools lets you write clearer, more efficient code. We will discuss Rust procedural macros and how they can change your code in this post.
What are Rust Macros?
Metaprogramming using Rust macros reduces boilerplate by letting you create code that writes other code. At build time, macros increase values, making them strong.
Rust has declarative and procedural macros. Pattern-based declarative macros are defined using macro_rules!. However, procedural macros let you build more complex, flexible code that generates Rust code from attributes or function-like syntax. This article discusses procedural macros and how they aid difficult tasks.
Understanding Procedural Macros
Rust procedural macros use the abstract syntax tree (AST) to build new code. A different crate defines them, and writers can use traits or syntax that looks like functions to write Rust code.
Use Cases
The creation of custom attributes for user-defined code or custom derive traits (like #[derive(Debug)]) are typically automated using procedural macros. In frameworks like Serde, procedural macros produce serialization and deserialization code for complicated data structures.
How it Works
The compiler uses procedural macros to produce code from input syntax. After inspecting and modifying the input code, the macro produces the required output, which is built alongside the application itself.
Writing Your First Procedural Macro
Step-by-step instructions for creating a basic procedural macro.
Step 1. Create a New Crate
First, develop a procedural macro crate. Run this in terminal:
cargo new hello_macro âlib
Add proc-macro to the Cargo.toml file to enable procedural macros:
[lib]
proc-macro = true
Step 2. Define the Macro
Inside lib.rs, define your first procedural macro. Here's an example that creates a hello_macro:
extern crate proc_macro;
use proc_macro::TokenStream;
#[proc_macro]
pub fn hello_macro(input: TokenStream) -> TokenStream {
// Return the code that should be generated
let output = "fn hello() { println!(\"Hello, macro!\"); }";
output.parse().unwrap()
}
Step 3. Apply the Macro
Now, in your main crate, add the following:
use hello_macro::hello_macro;
hello_macro!();
fn main() {
hello(); // Calling the generated function
}
Advanced Procedural Macro Concepts
Attribute Macros
Create custom code attributes using attribute procedural macros for increased versatility. Libraries like Serde produce struct serialization and deserialization code using attribute macros. Rust's macro system is strong and extendable because you can create attributes.
Token Streams
Procedural macros use Rust syntax token streams. Token streams enable programmatic manipulation of complicated code structures. This lets procedural macros build simple code and complex logic appropriate to your application.
Error Handling
Custom error handling is possible in Rust procedural macros. Make sure macros produce relevant compile-time warnings to help users use them correctly. You can use the syn crate to parse data and produce descriptive error messages if an attribute is invalid.
Conclusion
Rust procedural macros save boilerplate and allow advanced metaprogramming. They simplify tedious procedures and increase codebase flexibility by generating code at compile time. Procedural macros enable custom derives, error-free code, and plugin systems in Rust. Experiment and use Rust macros in your projects now!
313 views