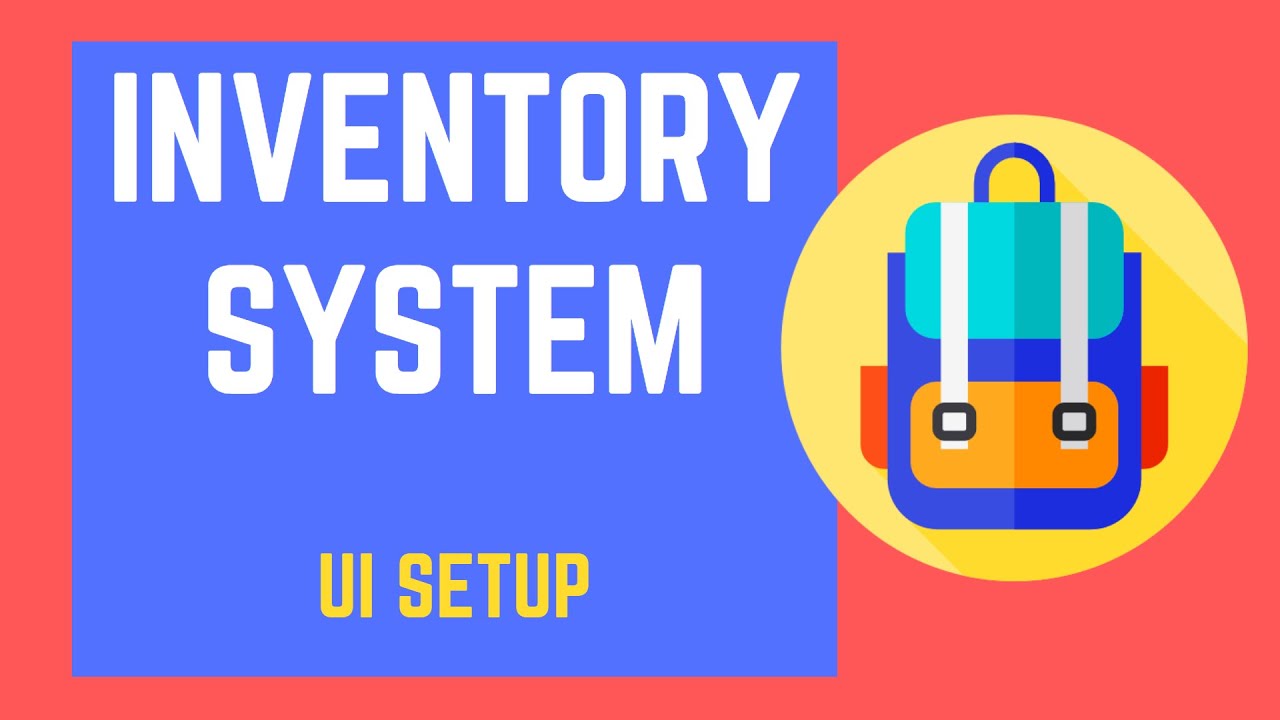
January 03, 2025
Designing an Inventory System for RPGs with UI Integration
When you play an RPG, have you ever thought about how the inventory system works? The ability to pick up, arrange, and use things adds dimension to any Role-Playing-Game. In this step-by-step tutorial, we will develop an RPG-style inventory system with UI components for picking up, dropping, and using items. Let's get started!
Setting Up the Inventory Structure
A game inventory system is just a collection of items. Each slot in an array or list in RPGs may store an object or be empty. Name, kind, and quantity are usually on each item. A basic inventory structure with a specified number of slots:
class Item:
def __init__(self, name, type, quantity=1):
self.name = name
self.type = type
self.quantity = quantity
class Inventory:
def __init__(self, size):
self.items = [None] * size # Initialize with empty slots
This simple design enables us set an inventory with many slots for different items. Add the functions to handle these things next.
Coding the Core Inventory Logic
After creating the inventory structure, write the logic for adding, deleting, and using items. How each function works:
- Add Item: Check inventory for any slot available. Increase the amount if the item exists; else, insert it in an empty slot.
- Remove Item: Find the item by index, lower its amount if stackable, or delete it if zero.
- Use Item: Activate the item's effect (e.g., healing) and reduce or eliminate it if used.
Here's some sample code to illustrate this:
class Inventory:
def __init__(self, size):
self.items = [None] * size
def add_item(self, item):
for i, slot in enumerate(self.items):
if slot and slot.name == item.name:
slot.quantity += item.quantity # Add to existing stack
return
elif slot is None:
self.items[i] = item # Place item in empty slot
return
def remove_item(self, index):
if self.items[index]:
if self.items[index].quantity > 1:
self.items[index].quantity -= 1
else:
self.items[index] = None
def use_item(self, index):
item = self.items[index]
if item:
print(f"Using {item.name}")
self.remove_item(index) # Use up one item
Creating the UI for the Inventory
Effective inventory systems require user interfaces so players can interact graphically. Adding, using, and removing items will update our inventory display.
- Display Item Slots: Set icons and titles for each slot and identify amount if stackable.
- Dynamic Updates: The UI should update instantly when an item is picked up or used.
This pseudocode shows a simple inventory UI setup:
def update_inventory_ui(inventory):
for index, item in enumerate(inventory.items):
if item:
print(f"Slot {index+1}: {item.name} x{item.quantity}")
else:
print(f"Slot {index+1}: Empty")
This function refreshes the display depending on item availability and amount for each slot. In a gaming engine, these print statements would be replaced with user interface icon and label images.
Adding Interaction: Picking Up, Dropping, and Using Items
Lastly, we need functions to connect with the items within the game:
- Pick Up: When the user interacts with game things, add them to the inventory.
- Drop Item: In this function, the item will be available for pickup in-game if the user drops it.
- Use Item: Users can use available items from inventory by clicking on them in their or using hotkeys.
def pick_up_item(inventory, item):
inventory.add_item(item)
def drop_item(inventory, index):
item = inventory.items[index]
if item:
print(f"Dropped {item.name}")
inventory.remove_item(index)
# Example interaction
pick_up_item(inventory, Item("Health Potion", "consumable", 1))
update_inventory_ui(inventory)
These capabilities let players interact with the inventory graphically and in-game, providing a dynamic, responsive system.
Conclusion
Building an RPG inventory system is challenging but provides depth and authenticity. We have covered inventory structure, coding the main logic for adding and using items, UI design, and player interactions for picking up and dropping stuff. You can use item categories and filters to create a customized inventory system that enriches your RPG experience!
1.5k views