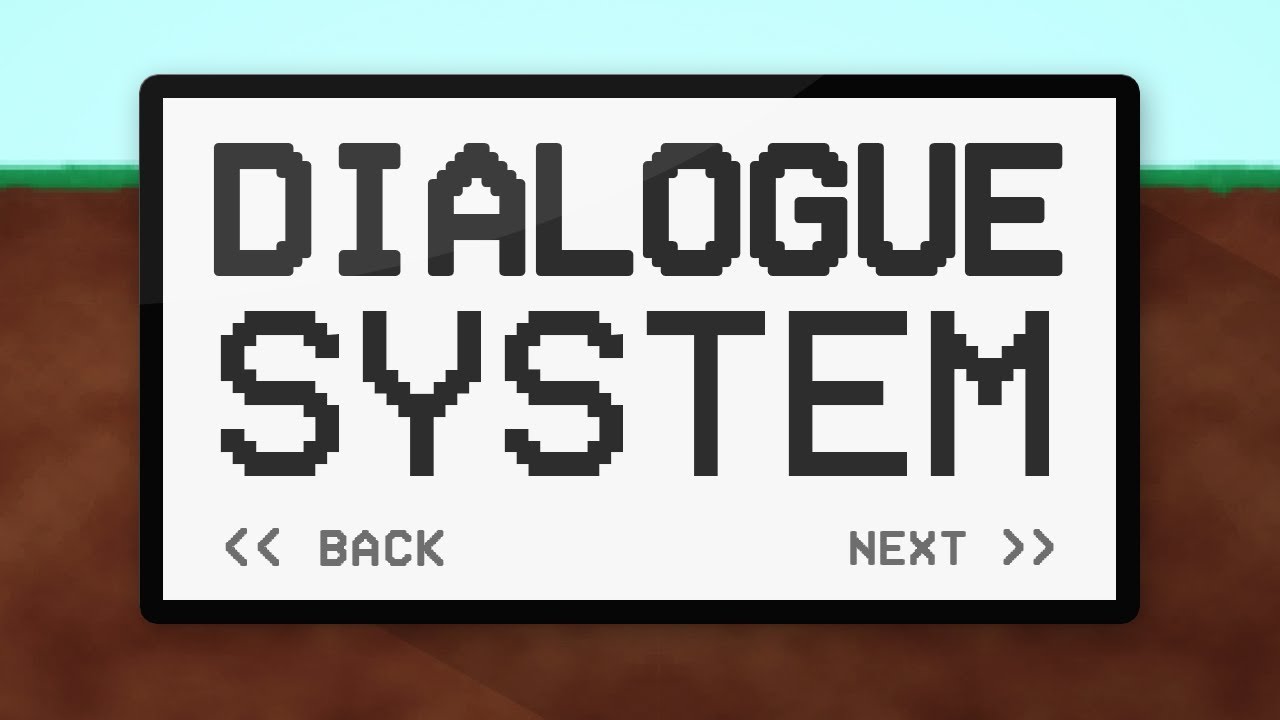
December 30, 2024
Coding a Simple Dialogue System for Narrative Games
Does a game's dialogue seem like a conversation that immerses you? Dialogue systems enable players engage with characters and the storyline in most narrative-driven games. A pleasant and functional dialogue system is crucial to your game's narrative, whether you include basic chat or a branching plotline. Let's learn how to develop a basic, powerful game dialogue system.
Choosing the Right Tools
Choose the correct game platform before developing. Unity and Unreal Engine provide scripting features that are good sources for conversation systems.
- Unity: C# makes Unity easy to implement with simple scripting.
- Unreal Engine: The Unreal Engine supports Blueprint for visual programming and C++ for advanced features. Choose the appropriate coding approach for your project based on your comfort level.
Unity is a simplistic example, but the ideas apply to Unreal Engine.
Basic Dialogue System Setup
Set up your conversation system's basic structure initially. You must manage dialogue lines data, including character names, text, and player options.
Unity supports this with arrays and lists. Here's an example of how to structure a simple dialogue line:
public class DialogueLine
{
public string characterName;
public string dialogueText;
}
Next, create a list to hold multiple lines of dialogue:
public List<DialogueLine> dialogueLines;
You can now save a whole string of dialogue for a conversation. Based on the player's game state, load this list dynamically.
Implementing Dialogue Flow
Now comes the fun, flowing conversation and player interaction. Control how dialogue develops, whether automatically or by player decision.
Here's some Unity C# code that shows the dialogue and lets the player make choices:
public class DialogueManager : MonoBehaviour
{
public Text dialogueText;
public List<DialogueLine> dialogueLines;
private int currentLine = 0;
void Start()
{
ShowDialogue();
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
currentLine++;
if (currentLine < dialogueLines.Count)
ShowDialogue();
else
EndDialogue();
}
}
void ShowDialogue()
{
dialogueText.text = dialogueLines[currentLine].characterName + ": " + dialogueLines[currentLine].dialogueText;
}
void EndDialogue()
{
dialogueText.text = "End of Dialogue";
}
}
Pressing spacebar moves the dialogue to the next line. You can add player options using a branching conversation tree that changes based on their choice.
Set up the dialogue flow in Unreal Engine using Blueprint nodes and Event Tick or Input Action nodes for advancement and text.
Adding Character Portraits and Audio
Character images and voiceovers improve dialogue systems. This gives conversations emotional depth and makes them more vibrant.
Displaying character photos in UI elements in Unity lets you shift visuals based on the speaker:
public Image characterPortrait;
void ShowDialogue()
{
dialogueText.text = dialogueLines[currentLine].characterName + ": " + dialogueLines[currentLine].dialogueText;
characterPortrait.sprite = GetCharacterPortrait(dialogueLines[currentLine].characterName);
}
Sprite GetCharacterPortrait(string characterName)
{
// Return the appropriate portrait based on the character name.
}
Use UMG (Unreal Motion Graphics) to show pictures and Audio Components for character voices in Unreal Engine.
Testing and Refining the Dialogue System
Playtest the system after setup. Make sure there is smooth dialogue and choices that count. Add situations that change dialogue based on player actions or choices to enhance the system. Meeting narrative pace requires timing and presentation adjustments.
Conclusion
A basic but effective dialogue system can make your narrative game more interesting and let players influence the story. Building your own dialogue system in Unity or Unreal Engine provides you full story flexibility. After learning the basics, try branching dialogues, dynamic answers, and custom animations for a realistic experience!
555 views