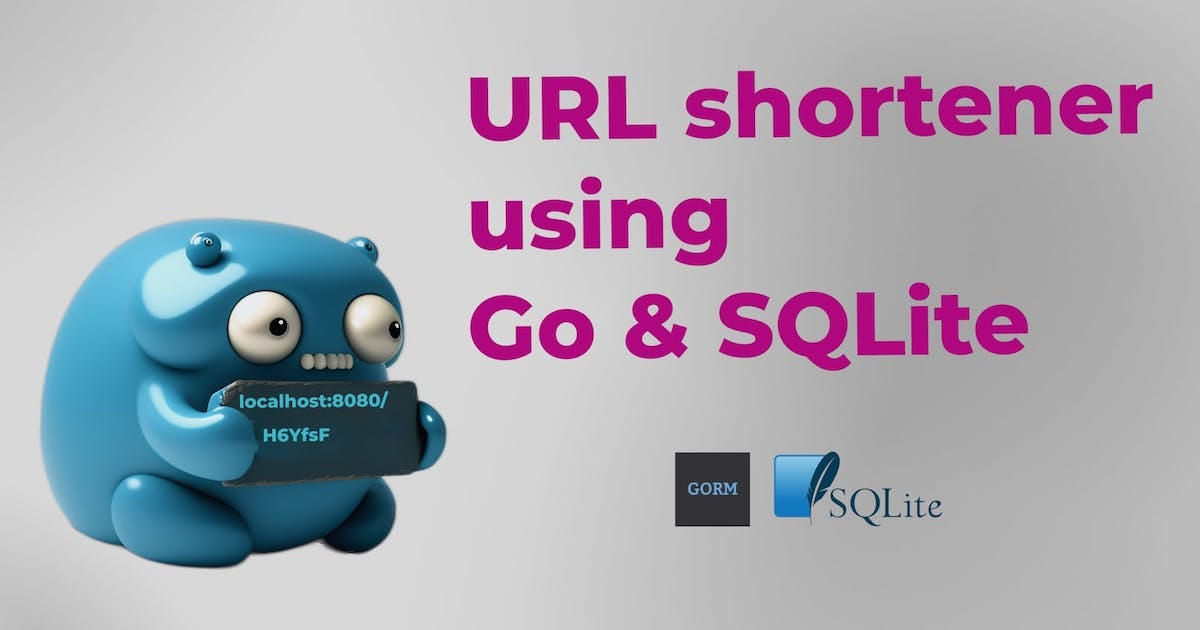
April 29, 2025
Create a Scalable URL Shortener with Go
We have all seen those shorten links on social media or in marketing efforts with weird characters. They monitor, share, and simplify lengthy URLs as well as provide ease. Creating a URL shortener for hundreds or millions of users? What a challenge.
This article will show you how to build a scalable URL shortener in Go (Golang), a fast and efficient programming language. After reading this guide, you will know how to develop a simple URL shortener and scale it for big traffic.
Putting Together the Go World
We will set up the development environment before developing the URL shortener. Start by downloading and installing Go from the official Go website. After installing Go, set up a new project by creating a directory and executing go mod init . This initializes Go modules and simplifies dependencies.
A common Go package that facilitates request routing is gorilla/mux. Install using go get github.com/gorilla/mux. Let's use PostgreSQL, although you may use any relational database.
Designing the URL Shortener
We should think about how our URL shortener looks for a moment. It works simply: users enter lengthy URLs, and the method creates shorter ones. A database stores this short URL, which sends users to the lengthy URL.
We need a table to hold short and long URLs. Table schema may look like this:
CREATE TABLE urls (
id SERIAL PRIMARY KEY,
short_url VARCHAR(255) NOT NULL,
long_url TEXT NOT NULL
);
Hashing will yield the short URL. Any method will work, however base62 encoding is common. This method generates compact short URLs using alphanumeric letters (0-9, A-Z, a-z). The system may create a 6-character URL like "abc123" instead of a 200-character one.
Building the Core API
Building the API is our project's core. This is easy in Go using gorilla/mux. Shortening URLs and redirecting them to their original form will be our main endpoints.
This Go code handles the URL shortening endpoint:
// Shorten URL handler
func shortenURL(w http.ResponseWriter, r *http.Request) {
var urlInput struct {
LongURL string `json:"long_url"`
}
err := json.NewDecoder(r.Body).Decode(&urlInput)
if err != nil {
http.Error(w, "Invalid request", http.StatusBadRequest)
return
}
// Generate short URL (use your encoding method here)
shortURL := generateShortURL(urlInput.LongURL)
// Save to database
db.Exec("INSERT INTO urls (short_url, long_url) VALUES ($1, $2)", shortURL, urlInput.LongURL)
json.NewEncoder(w).Encode(map[string]string{"short_url": shortURL})
}
When someone accesses a short URL, the redirect endpoint should search it up in the database and send them to the long URL. It could be done in this way:
// Redirect handler
func redirectToLongURL(w http.ResponseWriter, r *http.Request) {
shortURL := mux.Vars(r)["short_url"]
var longURL string
err := db.QueryRow("SELECT long_url FROM urls WHERE short_url = $1", shortURL).Scan(&longURL)
if err != nil {
http.Error(w, "URL not found", http.StatusNotFound)
return
}
http.Redirect(w, r, longURL, http.StatusFound)
}
These handlers allow us to take lengthy URLs from users and produce short URLs that redirect to the original links.
Handling Scalability
Scalability will become important as your URL shortener develops. In high-traffic conditions or if your service becomes viral, you need a system that can manage a lot of requests without crashing.
Horizontal scaling involves distributing your database over numerous servers. This may prevent server bottlenecks. A Redis caching layer can handle frequent brief URL lookups. Caching previously viewed short URLs may greatly decrease database demand and speed up redirection.
Shard your database to distribute load over numerous databases based on hash ranges for long-term use. This will strengthen your system against increased traffic.
Deploying the URL Shortener
You must deploy the app after building it. Docker makes Go app containerization easy. Docker lets you execute the app reliably in local or cloud environments. Start with AWS, DigitalOcean, or Google Cloud for deployment.
Configure CI and CD pipelines to automate testing and deployment. GitHub Actions, GitLab CI, and Jenkins can speed development and deploy changes.
Conclusion
Building a scalable URL shortener in Go is a great approach to learn about its web development and high-traffic application capabilities. We addressed URL shortening and redirection, scalability, and deployment techniques. To make your URL shortener more useful, you can add user accounts, data, and other things with these tools.
This guide should help you create and scale a URL shortener for real-world application.
76 views