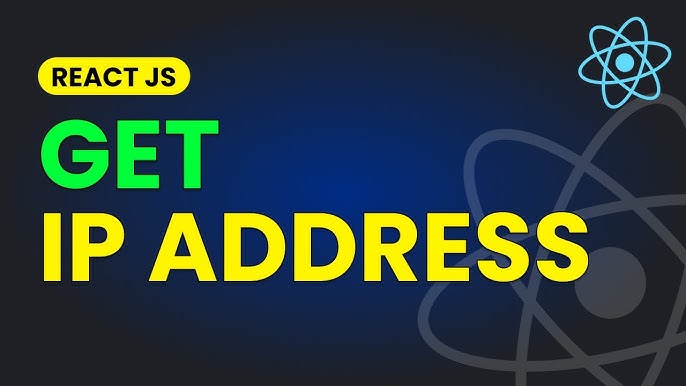
April 28, 2025
Build an IP Address Finder with React in Minutes
Ever thought about your IP address? You may be troubleshooting a network or investigating your digital footprint. Whatever the reason, an IP address finding tool is surprisingly handy, and we will create one in minutes using React.
React, the popular JavaScript library for designing dynamic user interfaces, is ideal for our project. React's simplicity and a free API will let us build an IP address finder quickly. So let's jump in!
Prerequisites
You will need a few things before we get started. React users are ahead of the curve. If not, no worries! Keep things simple. Install Node.js and npm (or yarn). Finally, we will get IP address data via a free API like ipify or ip-api.
Setting Up Your React Project
The first thing we need to do is make a new React app. This is how you set one up if you do not already have one:
In your terminal, run:
npx create-react-app ip-finder
This command creates a brand-new React app named ip-finder. Do that first, then go to the project folder:
cd ip-finder
Now let's install Axios, a library that will help us send HTTP requests:
npm install axios
It is easy to use and powerful, which is great for our API calls to get the IP address. We are now ready to start writing code!
Building the IP Address Finder Component
It is now time to build the IpFinder component, which is the heart of our app. We will use useState in React to handle the IP address info and useEffect to make the API call when the component starts.
Within the src folder, make a new file named IpFinder.js. This is the code you need:
import React, { useState, useEffect } from "react";
import axios from "axios";
const IpFinder = () => {
const [ip, setIp] = useState("");
useEffect(() => {
const fetchIp = async () => {
try {
const response = await axios.get("https://api.ipify.org?format=json");
setIp(response.data.ip);
} catch (error) {
console.error("Error fetching IP address:", error);
}
};
fetchIp();
}, []);
return (
<div>
<h1>Your IP Address</h1>
<p>{ip ? ip : "Loading..."}</p>
</div>
);
};
export default IpFinder;
The empty string ("") is used to start the useState hook, which holds the IP address. It is changed when the API call returns the data. When the component starts, the useEffect hook makes an API call. This is a great place for side effects like getting data. It sends a GET request to https://api.ipify.org?format=json and gets back the public IP address in JSON format. The data is shown inside a
tag once it has been properly fetched. The text will say "Loading..." until the IP address is found if the data is still being loaded.
Integrating the Component into the App
After making the IpFinder part, it is time to add it to our main App.js file. Change the following in the App.js file:
import React from "react";
import IpFinder from "./IpFinder";
function App() {
return (
<div>
<IpFinder />
</div>
);
}
export default App;
We tell React to show our IP address finder when the app loads by importing IpFinder and using it inside App.
Testing the App
Now that we've got the app set up, let's use it! You can run the app by:
npm start
This will start the development server. To use it, go to http://localhost:3000 in your browser. In the browser, your public IP address should show up. There should be no problems, and it should appear in a few seconds. Do not worry if the IP address is still loading; it is just waiting for the API response.
Enhancements and Next Steps
We can always make our IP Address Finder better, even though it works perfectly but enhancements are never ending. Here are some ways to make it better:
- Geolocation data: To show where the IP address is located, such as the city, country, and timezone, use a different API, such as ip-api.
- Styling: The app looks simple right now, but it is easy to make it look nice with CSS or a UI system like Material-UI or Tailwind CSS.
- Dynamic refresh: Add a button that updates the IP address so users can see changes right away without having to restart the page.
- Deployment: Are you ready to share your tool? If you put it on a site like Netlify or Vercel, it will be live in minutes.
Conclusion
And in just a few minutes, you have made an IP address finder with React and Axios. This was easy to do because React is simple and flexible, and Axios handled the API calls well. You can now easily verify your IP address with this app or add functionality as needed.
The best part? You now have a realistic React app that works. This project is a great way to improve your skills as a worker, no matter how much experience you have. Feel free to change it, share it, and make it your own!
401 views