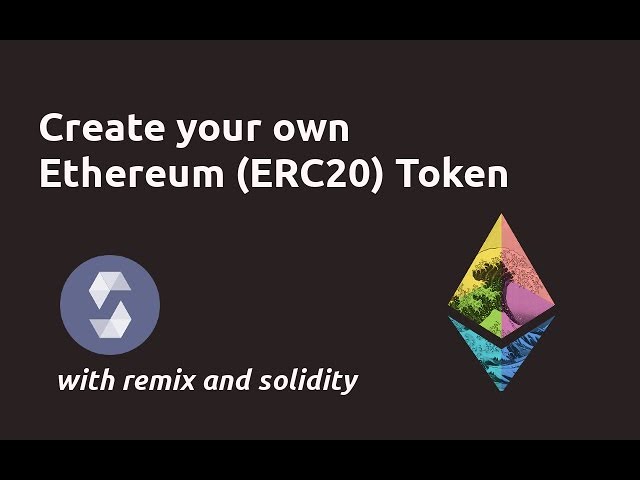
March 10, 2025
Creating Your Own Cryptocurrency Token on Ethereum: A Beginner's Guide
Have you considered making your own cryptocurrency? Blockchain technology makes Ethereum bespoke token creation simpler than ever. Creating an ERC-20 token is a great place to start for developers and crypto fans alike. This blogpost explains how to code and deploy an Ethereum-based cryptocurrency token using Solidity.
What is an ERC-20 Token?
Knowing what an ERC-20 token is important before writing code. ERC-20 is an Ethereum blockchain smart contract standard. To be compatible with Ethereum, tokens must meet its rules. These guidelines make the token compatible with wallets, exchanges, and dApps.
ERC-20 tokens represent assets, facilitate transactions, and power DeFi systems. Tokens that are ERC-20 are the most well-known cryptocurrency, such as USD Coin (USDC) and Chainlink (LINK).
Setting Up Your Development Environment
Code writing requires the right tools. Creating and deploying an ERC-20 token requires:
- Solidity: The programming language for Ethereum smart contracts.
- Remix IDE: A web-based developer tool for Solidity.
- MetaMask: A browser plugin/extension for Ethereum blockchain interaction.
- Use Ethereum Test Networks: Like Rinkeby or Ropsten to avoid spending actual Ether during development.
After installing and configuring these tools, you may write your smart contract.
Writing the ERC-20 Token Smart Contract
Let's write the smart contract. The contract will define your token's name, symbol, supply, and user interaction.
Here's a simple example of an ERC-20 token written in Solidity:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract MyToken {
string public name = "MyToken";
string public symbol = "MTK";
uint8 public decimals = 18;
uint256 public totalSupply;
mapping(address => uint256) public balanceOf;
mapping(address => mapping(address => uint256)) public allowance;
constructor(uint256 _initialSupply) {
totalSupply = _initialSupply * 10**uint256(decimals);
balanceOf[msg.sender] = totalSupply;
}
function transfer(address recipient, uint256 amount) public returns (bool) {
require(balanceOf[msg.sender] >= amount, "Insufficient balance");
balanceOf[msg.sender] -= amount;
balanceOf[recipient] += amount;
return true;
}
function approve(address spender, uint256 amount) public returns (bool) {
allowance[msg.sender][spender] = amount;
return true;
}
function transferFrom(address sender, address recipient, uint256 amount) public returns (bool) {
require(balanceOf[sender] >= amount, "Insufficient balance");
require(allowance[sender][msg.sender] >= amount, "Allowance exceeded");
balanceOf[sender] -= amount;
balanceOf[recipient] += amount;
allowance[sender][msg.sender] -= amount;
return true;
}
}
Deploying Your ERC-20 Token
After creating your smart contract, deploy it on Ethereum. The quickest approach to deploy the contract is utilizing Remix IDE to compile and deploy code straight to the blockchain.
- Compile the Code: To compile the code in Remix, pick the "Solidity Compiler" tab and click "Compile."
- Connect MetaMask: Install and setup MetaMask for a test network (e.g. Rinkeby) and connect it to Remix.
- Deploy Contract: Select contract in "Deploy & Run Transactions" tab and click "Deploy." MetaMask requires transaction confirmation.
- Verify Deployment: Search Etherscan for the contract address to verify deployment.
Conclusion
Ethereum token creation is fun and rewarding. Start your own ERC-20 token on the blockchain, open up endless possibilities, and explore DeFi applications with these simple steps. You can generate a token rapidly with the right tools and coding skills!
241 views