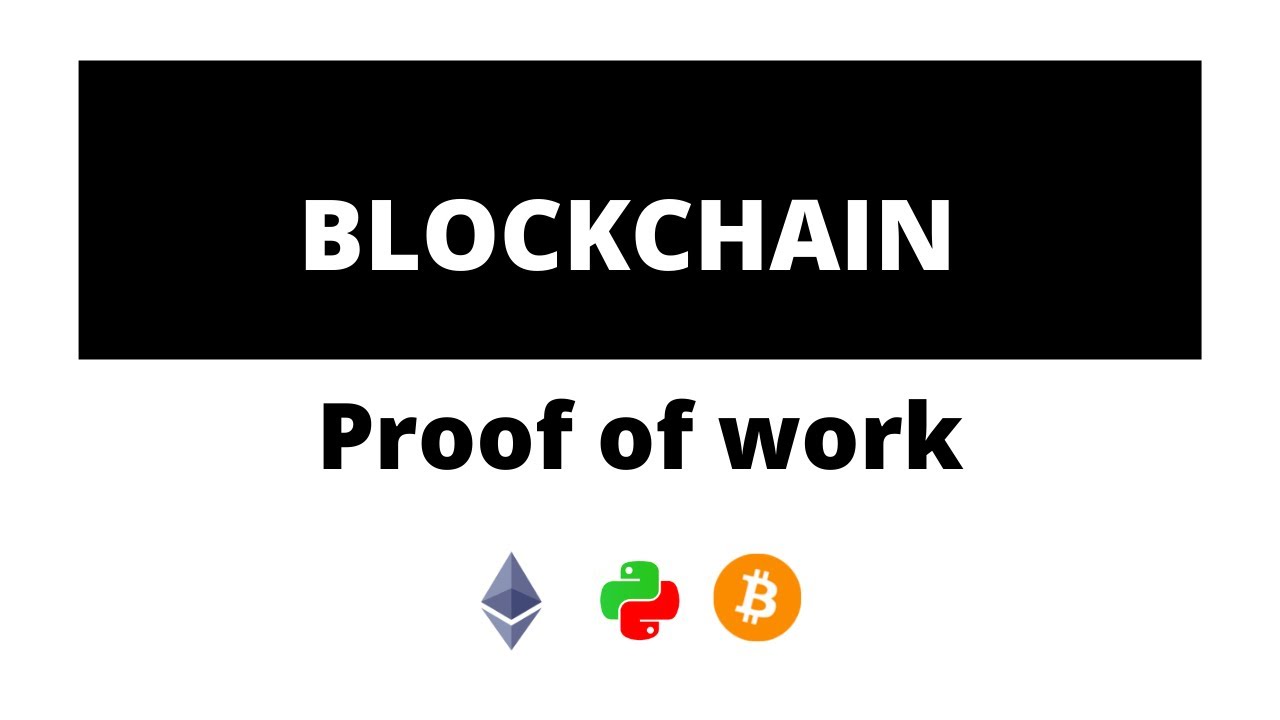
Wondering how Bitcoin is secure without a central authority? Proof of Work (PoW), a vital mining technique, protects blockchain network stability. Decentralized currencies are safe, fraud-free, and network-efficient, thanks to Proof of Work.
We will describe PoW's structure and effectiveness in this blogpost. To help you understand this interesting topic, we will walk you through building a basic PoW algorithm in Python.
Understanding the Mining Process
Mining validates and adds blockchain transactions, producing Proof of Work. First, to answer difficult math problems, add the block to the chain and get money.
This approach requires one-way cryptographic hash algorithms that convert input data into fixed-size outputs. Miners use a changing nonce to hash the block's transaction information and the preceding block's hash in PoW. Your nonce should yield a hash with a set number of leading zeros.
It takes computational effort to obtain the proper nonce, proving the miner's efforts.
The Mechanics of Proof of Work
Proof of Work makes valid hash harder. While it is trivial to calculate a hash from block data, obtaining a valid one with the right starting zeros takes time. Bitcoin adds new blocks every 10 minutes by adjusting its difficulty.
The network is secure because of difficulties. More difficult if a miner discovers a valid nonce too soon, less if too slow. This keeps the process difficult and maintains blockchain integrity.
Implementing PoW in Python
Let's construct a simple Proof of Work algorithm in Python. It will explain how miners find a valid nonce and how hashing works.
Step 1: Importing Required Libraries
Python's hashlib library makes computing cryptographic hashes like SHA-256 easy.
import hashlib
Step 2: Define the Block Structure
This simple implementation defines a block structure containing index, previous block hash, data, and nonce. We will include simple features for simplicity. However a true blockchain has complex structures.
class Block:
def __init__(self, index, previous_hash, data):
self.index = index
self.previous_hash = previous_hash
self.data = data
self.nonce = 0
self.hash = self.calculate_hash()
def calculate_hash(self):
block_string = str(self.index) + self.previous_hash + str(self.data) + str(self.nonce)
return hashlib.sha256(block_string.encode('utf-8')).hexdigest()
Step 3: Proof of Work Function
PoW relies on mining, where we repeatedly hash the block's contents with varying nonce values until we obtain a hash with the desired amount of leading zeros.
def proof_of_work(block, difficulty):
target = '0' * difficulty # Target difficulty (e.g., '0000')
while block.hash[:difficulty] != target:
block.nonce += 1
block.hash = block.calculate_hash()
return block
Step 4: Running the PoW Algorithm
Let us execute the PoW algorithm on a sample block with a difficulty of 4 (the hash should start with four zeros).
block = Block(1, '0', 'Sample Block Data')
difficulty = 4
print("Mining block...")
mined_block = proof_of_work(block, difficulty)
print(f"Block mined: {mined_block.hash}")
Running this code will change the nonce value until it finds a hash with four leading zeros, demonstrating the computing work necessary for mining.
Testing and Output
Upon running the code, youââ¬â¢ll see output like the following:
Mining block...
Block mined: 000048e9e9f2f82e38c9a2d09b290f4ec631b4eb7d3427a6b5a4d0ab8d31ed27
This result shows how the nonce fluctuates throughout mining, and the final hash contains four leading zeros.
Conclusion
Proof of Work is a crucial technique for blockchain security and decentralization. PoW restricts block addition to miners who solve computational challenges. This basic Python code shows how this process works, helping you comprehend Bitcoin's complexity.
The PoW algorithm underpins blockchain technology and affects cybersecurity and decentralized systems beyond money. This simple implementation helps you understand blockchain's security and integrity.
248 views