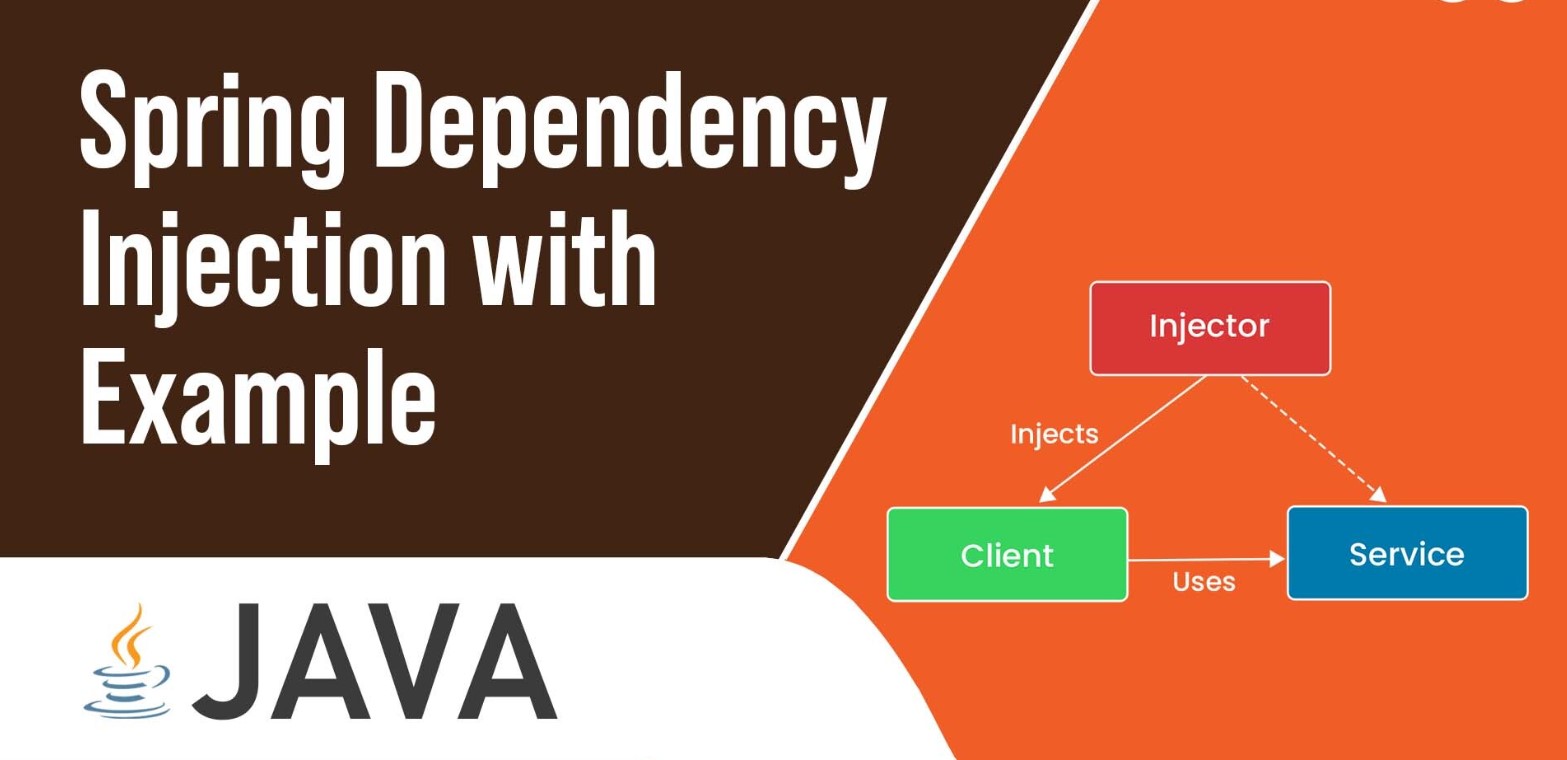
February 11, 2025
Understanding Dependency Injection in Spring: Simplifying Component Management
Ever battled with app object dependencies? Imagine manually building and maintaining class connections as your project grows. It may quickly become a nightmare! Due to its Dependency Injection (DI) architecture, Spring Framework component maintenance is easy. This article explains DI, how it works in Spring, and how to use it to write clean, maintainable code.
What is Dependency Injection?
Dependency Injection (DI) is a design paradigm that injects dependencies instead of constructing them. It reduces application component coupling.
In basic words, a framework like Spring provides a class with its dependencies instead of having it construct them. There are two main DI types:
- Constructor Injection: Class constructor provides dependencies.
- Setter Injection: Add dependencies after object formation via setters.
Why Use Dependency Injection in Spring?
Tight coupling between classes makes them hard to adapt, test, or scale using traditional object management. Changes to one class could affect the whole program. DI allows component replacement or update without changing dependent classes.
Spring's DI container handles bean and dependency lifecycles. You simply need to establish component relationships; Spring does the rest. This method saves boilerplate code, improves scalability, testability, and flexibility, particularly in large systems.
Implementing DI in Spring
An example will demonstrate how to build Dependency Injection in a Spring application. Imagine we have Car and Engine classes. The Car class needs the Engine class to work.
Step 1: Set up a basic Spring project
Spring supports XML and Java-based DI setup. We will utilize annotation-based Java setup for simplicity.
Step 2: Create the components (Beans)
// Engine class (Dependency)
public class Engine {
public void run() {
System.out.println("Engine is running!");
}
}
// Car class (Dependent on Engine)
public class Car {
private Engine engine;
// Constructor Injection
public Car(Engine engine) {
this.engine = engine;
}
public void start() {
engine.run();
}
}
Step 3: Inject dependencies using constructor or setter methods
Spring's DI lets us inject Engine into Car's constructor.
// Spring configuration using annotations
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// Spring automatically injects Engine into Car via constructor
}
The @ComponentScan annotation instructs Spring to search the package for Car and Engine components. Spring generates the beans and injects the Engine instance into the Car bean.
DI Annotations in Spring
Spring provides many annotations to simplify DI:
- @Autowired: Spring automatically injects beans into target classes via constructor or setter methods.
- @Component: Identifies a class as a Spring component, enabling automated detection and lifecycle management.
- @Configuration: Indicates that the class has Spring configuration information.
- @Bean: Allows manual control over bean generation in Java-based setup.
Spring creates and injects dependencies effortlessly using these annotations, letting you concentrate on business logic instead of writing extra code.
Advantages of Dependency Injection in Spring
There are many ways in which DI enhances the design and maintainability of applications:
- Loose Coupling: DI loosens coupling, making classes easier to update and test. Code becomes modular and clearer.
- Improved Testability: DI enhances testability by simplifying unit testing. Injecting fake dependencies lets you test components without caring about their dependencies.
- Flexibility and Maintainability: DI provides flexibility and maintainability by allowing for alternative implementations without impacting dependant classes. Swapping components without altering the program is easy.
To change the Engine class of the Car class to a new engine type, you simply need to change the configuration.
Conclusion
Spring Dependency Injection simplifies object dependency management, making code more flexible, manageable, and testable. DI lets you decouple components, making your project more scalable and flexible. Spring's DI container simplifies dependency management for small projects and big applications. Why not explore DI and use it in your Spring apps to get its full benefits?
281 views