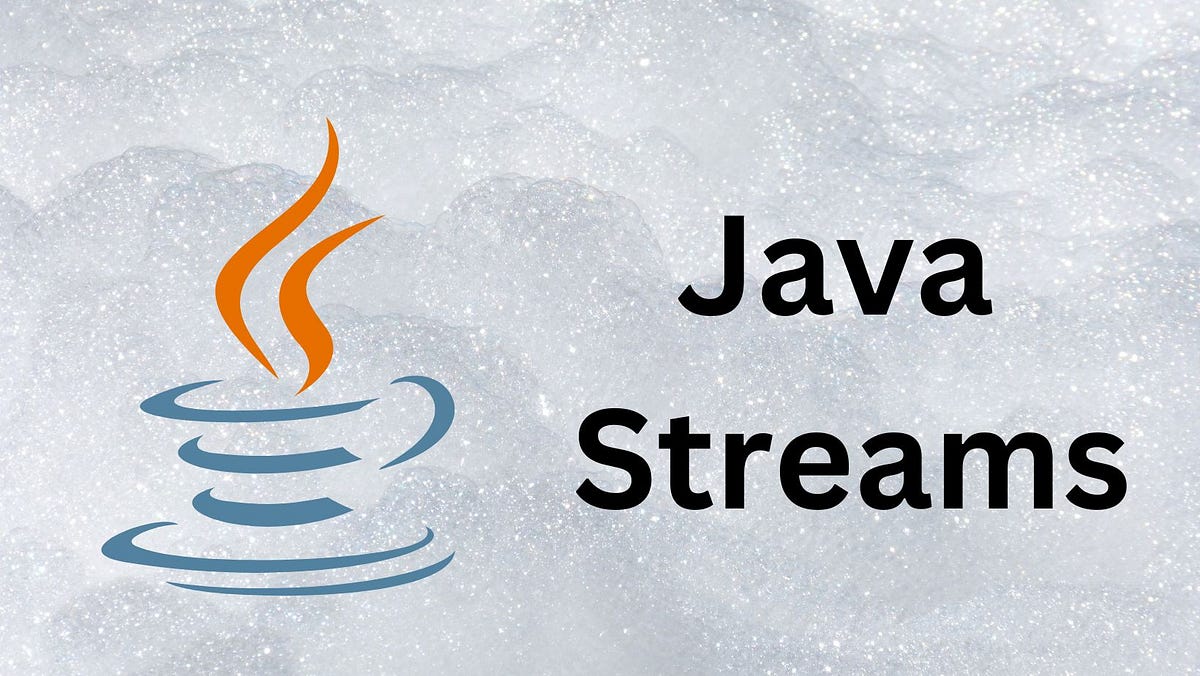
Have you ever written long and endless Java loops to manage data? A more efficient, brief, and modern approach to handle collections? Java Streams, introduced in Java 8 has changed everything. Streams provide pipeline-like data processing, minimizing boilerplate code and improving readability and speed.
Let's discover how Streams can easily filter, map, and reduce data.
What Are Java Streams?
Java Streams provide efficient data processing. Streams use data pipelines to change, filter, and aggregate data, unlike collections.
Collections are data storage structures like ArrayList or HashSet, whereas streams handle data on demand. Streams are ideal for complicated processes like filtering and aggregation without intermediary storage.
Common Stream Operations
Data management is powerful using Java Streams. In particular, these three stand out:
- Filtering: Removes unwanted elements depending on a condition.
- Mapping: Transforms stream elements into new forms.
- Reducing: Combines data into a single result, such as a sum or maximum.
These operations and Streams' functional design allow brief and expressive data processing. Letâs discuss these operations with coding examples:
1. Filtering
For example, to filter even integers/numbers from a list:
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
List<Integer> evens = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evens); // Output: [2, 4]
Here, the filter accepts a predicate and returns a new Stream with just the entries that meet the condition.
2. Mapping
For example, to convert a list of names to uppercase:
List<String> names = List.of("alice", "bob", "carol");
List<String> upperNames = names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(upperNames); // Output: [ALICE, BOB, CAROL]
Map runs a function on each element to create a modified Stream.
3. Reducing
For example, to calculate the sum of a list of integers:
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println(sum); // Output: 15
Reduce repeats an operation from a starting value to combine elements.
Benefits of Using Streams
Why use Java Streams? Here are some strong reasons:
- Concise and Readable: Stream operations simplify coding by eliminating verbose loops and if-else expressions.
- Parallel Processing: Multicore computers can process streams in parallel, improving performance for large datasets.
- Boilerplate Reduction: Simplifies complicated data conversions with fewer lines of code.
Limitations and Best Practices
Limitations
- Streams are single-use: Streams are non-reusable once consumed.
- Functional paradigm: New Java 8+ users can find the functional paradigm challenging.
Best Practices
- Keep it simple: Minimize unnecessary chaining for readability.
- Use parallel streams wisely: Suitable for computationally intensive jobs but may cause overhead for lesser datasets.
- Select appropriate terminal operations: Such as collect for lists or forEach for iteration, to fulfill objectives.
Conclusion
Power and simplicity make Java Streams redefine data processing for developers. Filtering, mapping, and reducing allow you to develop beautiful, functional code that is efficient and readable.
Next time you manage a Java collection, try Streams. Their sleek and effective data processing tools may become your favorites!
406 views