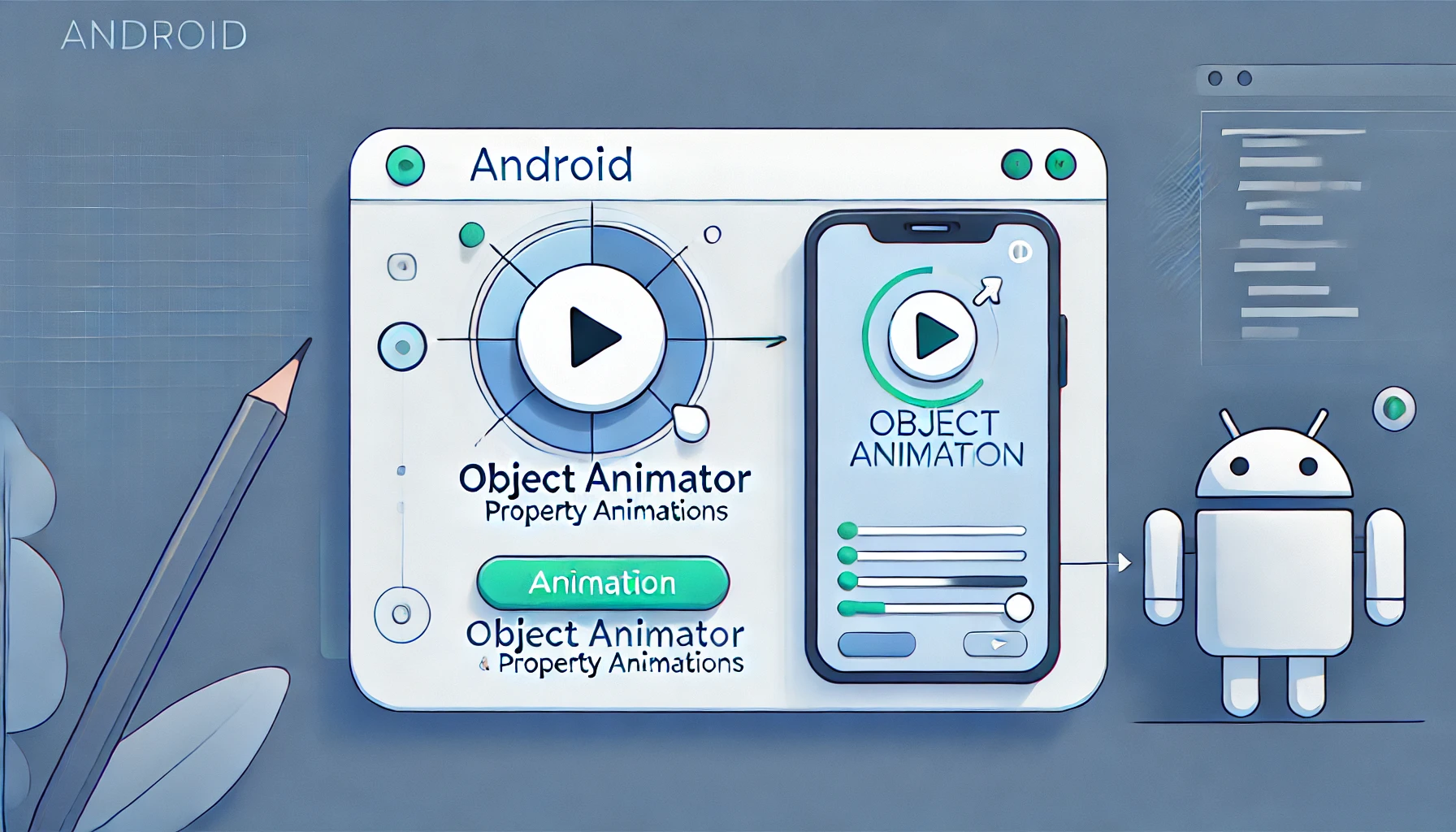
January 28, 2025
Animating Views with ObjectAnimator and Property Animations
Do you want to make your Android app more interactive? Animations are the key to making a simple application interesting and engaging. I will show you how to scale, rotate, and translate views using Android's ObjectAnimator and Property Animations.
Understanding ObjectAnimator and Property Animations
You must understand Android's Property Animation method before starting coding. Property Animations in API level 11 (Android 3.0) enable developers animate object characteristics like alpha, rotation, translationX, scaleX, and more. Property Animations are smoother and more configurable than ViewAnimation.
Property Animations use ObjectAnimator for view properties. Animation of a view's position, size, or rotation using a few lines of code creates dynamic user interfaces.
Setting Up ObjectAnimator
First, we will need to install ObjectAnimator. Basic syntax includes defining the view, property, and target values to animate. An example of horizontal screen translation:
ObjectAnimator animator = ObjectAnimator.ofFloat(view, "translationX", 0f, 500f);
animator.setDuration(1000);
animator.start();
ObjectAnimator is ideal for basic animations since it seamlessly transitions views with minimum coding.
Animating View Scaling
To emphasize a button, transition, or interaction effect, scaling a display can radically change its look. How to animate view scaling:
ObjectAnimator scaleX = ObjectAnimator.ofFloat(view, "scaleX", 1f, 2f);
ObjectAnimator scaleY = ObjectAnimator.ofFloat(view, "scaleY", 1f, 2f);
AnimatorSet animatorSet = new AnimatorSet();
animatorSet.playTogether(scaleX, scaleY);
animatorSet.setDuration(1000);
animatorSet.start();
Scaling gives your UI depth and emphasis by making it interactive. It is ideal for buttons, photos, and other highlights.
Animating Rotation
Rotation animations may make views lively. Rotating a view indicates engagement or a process (like a loading spinner). To rotate a view:
ObjectAnimator rotate = ObjectAnimator.ofFloat(view, "rotation", 0f, 180f);
rotate.setDuration(1000);
rotate.start();
Rotation animations not only enhance user experience but can also symbolize progress or indicate a change in state (such as in game development or interactive UIs).
Animating Translation
One of the most typical animations is smooth view movement. Translation animations are simple to use for button slides and game character movements. Example of horizontal view translation:
ObjectAnimator translate = ObjectAnimator.ofFloat(view, "translationX", 0f, 300f);
translate.setDuration(1000);
translate.start();
The view will slide along the X-axis here. Combining translation animations with scaling or rotation creates more sophisticated visual effects.
Conclusion
Dynamic animations for Android views using ObjectAnimator and Property Animations make your app more interesting. Animations that resize buttons, rotate symbols, and shift views across the screen are simple to create and enhance user experience. To enhance app animations and UI, experiment with settings and durations!
282 views