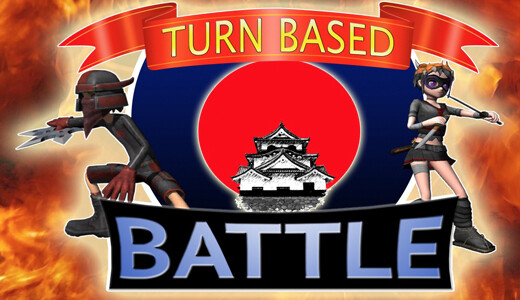
January 09, 2025
Building a Turn-Based Combat System for Strategy Games
How do Pokémon and Fire Emblem make every step tactical? Due to turn-based combat systems, players can strategically prepare each attack, defense, or healing spell. This article covers player and opponent turns, health points, and attack mechanisms for creating a turn-based combat system from scratch. By the end, you will know how to create strategic fights!
Key Elements of a Turn-Based Combat System
An excellent turn-based combat system uses turns, health points, and attacks. Players may critically evaluate each move with these aspects, adding complexity to gaming. When a person and an opponent switch roles, it gives both of them a chance to defend, fight, or heal. Health Points (HP) show how healthy a character is, while attack mechanisms show how much damage they do. You can play many turn-based games with just these three basic parts.
Setting Up the Code Environment
Our starting language is Python. Its simplicity makes even the simplest ideas clear. Python.org hosts it. For combat's unpredictability, we will use Python's random module to generate attack numbers.
Create a Python file to develop your turn-based system after setting up your environment.
Coding the Core Combat Loop
We will break down a basic turn-based battle loop's essential code. In this configuration, player and opponent characters have health points and exchange turns attacking until one is dead.
import random
# Player and enemy stats
player_hp = 100
enemy_hp = 100
# Simple attack function
def attack(attacker_name, defender_hp):
damage = random.randint(10, 30) # Random attack damage
print(f"{attacker_name} attacks for {damage} damage!")
return defender_hp - damage
# Main game loop
while player_hp > 0 and enemy_hp > 0:
# Player's turn
enemy_hp = attack("Player", enemy_hp)
print(f"Enemy HP: {enemy_hp}")
if enemy_hp <= 0:
print("Enemy defeated!")
break
# Enemy's turn
player_hp = attack("Enemy", player_hp)
print(f"Player HP: {player_hp}")
if player_hp <= 0:
print("Player defeated!")
break
Code Breakdown
Player and Enemy Health Points: In this simple turn-based combat system we've started both players and enemies with 100 HP. Damage decreases this amount each round until one character has no HP.
- Turns: The while loop continues as long as both characters have HP. The player hits first, then the adversary, in each loop. If either character's HP drops to 0 after each hit, the game is over.
- Attack Mechanics: The attack feature deals random damage between 10 and 30 points. This randomness is like fighting in real life, where hits do different amounts of damage.
This code makes a simple turn-based battle system that has big effects and results that you can not guess.
Enhancements and Strategy
After making the basic strategy, make it better! You might want to add special moves, attack types, or healing magic. The player can choose between a "normal attack" and a "heavy attack," which might miss but does more damage. You could also use a turn-order system or a stopping system that cuts damage in half. New elements complicate strategy, forcing players to plan their actions.
Characters can also get physical bonuses or protection scores to manage particular harm. Because of this added technique, players must thoroughly review each step.
Conclusion
For a turn-based battle system, this guide talked about setting up rounds, keeping track of health points, and writing basic attack mechanics. This foundation lets you create a complex, strategic combat system. So, are you ready to experiment and add your own twists to make your game battles more exciting and complicated?
270 views